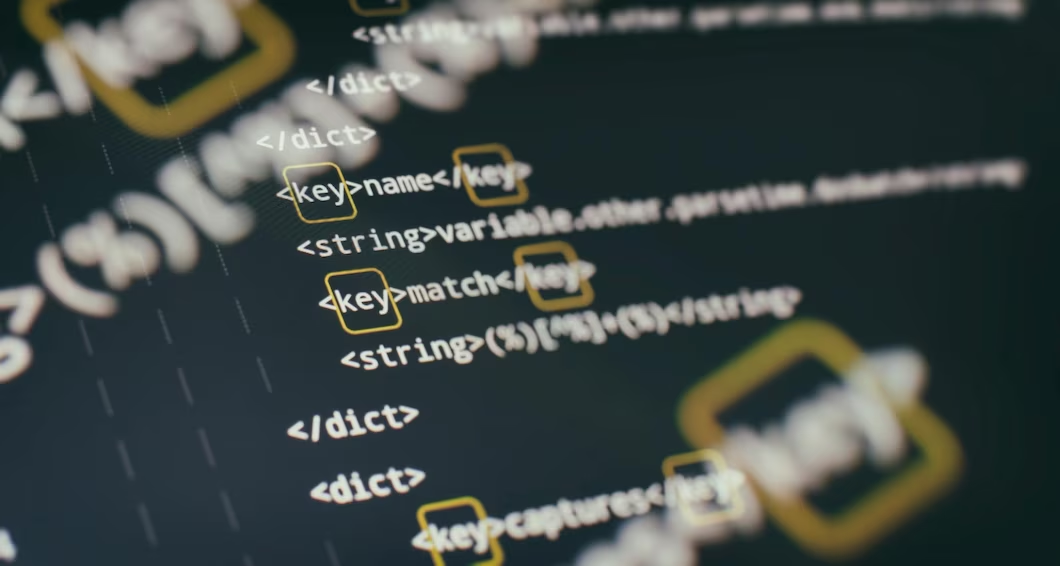
Journey with me into the intriguing world of web creation where JavaScript rules as a key protagonist. Envision JavaScript, like an artist with a brush, adding vivid classes to the monochrome canvas of HTML elements. This not only infuses life into those elements but also gifts them the ability to alter their demeanor and attire dynamically.
Shall we delve into the labyrinth of JavaScript to unearth the tricks it uses to masterfully add these classes? Steer your curiosity to these uncharted methods:
- .className;
- .classList.add(), and;
- setAttribute().
Ready to weave our way through the mysteries of each of these intriguing methods? Let’s embark on this exhilarating adventure.
1. The .className Method
The .className method is a JavaScript property that is used to set or retrieve the class names of an HTML element. It belongs to the Element interface of the Document Object Model (DOM), which provides a dynamic representation of a web page, enabling scripts to access and manipulate the page’s content, structure, and styles.
To understand how the .className method works, let’s consider an example HTML element with the id “myElement”:
<div id="myElement">Hello, World!</div>
If we want to add a class to this element using JavaScript, we can do so by using the .className property:
var element = document.getElementById("myElement");
element.className = "newClass";
In the above code snippet, we retrieve the element with the id “myElement” using the document.getElementById method, and then assign the value “newClass” to the className property of the element. As a result, the “newClass” class is added to the HTML element. It’s important to note that using this method will replace any existing classes on the element. Therefore, if the element had a previous class, it would be replaced entirely by “newClass”.
The .className method in JavaScript allows us to manipulate the class names of HTML elements dynamically. It provides a way to add, replace, or retrieve class names for an element, contributing to the dynamic styling and behavior of web pages.
2. The .classList.add() Method
The .classList.add() method is a JavaScript method that allows you to add one or more classes to an HTML element’s class attribute. It is part of the DOMTokenList interface, which represents a collection of space-separated tokens. Unlike the .className property, the .classList.add() method does not replace existing classes, allowing you to add multiple classes to an element without losing the previous ones.
To demonstrate the usage of the .classList.add() method, consider the following example:
var element = document.getElementById("myElement");
element.classList.add("newClass");
In this code snippet, we first retrieve the element with the id “myElement” using the document.getElementById method. Then, we call the .classList.add() method on the element and pass “newClass” as the argument. As a result, the “newClass” is added to the class attribute of the HTML element, without affecting any existing classes.
This method is particularly useful when you want to add or toggle classes dynamically based on certain conditions or user interactions. By leveraging the .classList.add() method, you can easily manipulate the classes of HTML elements, enabling dynamic styling and behavior in your web page.
The .classList.add() method provides a convenient way to add classes to an element’s class attribute without replacing existing classes. It enhances the flexibility and modularity of your JavaScript code by allowing you to modify the styling and behavior of HTML elements dynamically.
3. The setAttribute() Method
The setAttribute() method in JavaScript allows you to add or modify attributes of an HTML element. While it is not specifically designed for manipulating classes, it can also be used to add or update class attributes. This method takes two parameters: the name of the attribute and its corresponding value.
Consider the following code snippet:
var element = document.getElementById("myElement");
element.setAttribute("class", "newClass");
In this example, we retrieve the element with the id “myElement” using document.getElementById, and then use the setAttribute() method to set the class attribute to “newClass”. If the class attribute already exists, the setAttribute() method will update its value. If the attribute does not exist, it will create a new class attribute with the specified name and value.
However, it is important to note that using setAttribute() to modify the class attribute will overwrite any existing classes on the element. Therefore, if the element had other classes defined, they will be replaced entirely by the new class.
While the setAttribute() method is not specific to manipulating classes, it can be used to add or modify the class attribute of an HTML element. It provides a way to dynamically set or update attributes, including classes, on elements in a web page.
Detailed Application of Methods
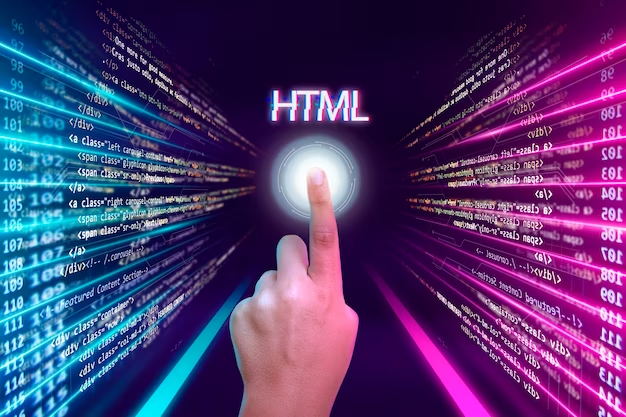
While the above examples show the basic usage of these methods, we need to dive deeper to understand their full range of applications.
Adding Multiple Classes
Both the .classList.add() and setAttribute() methods can be used to add multiple classes to an HTML element. Here’s how you can use each method:
- .classList.add(): Using the .classList.add() method, you can add multiple classes to an element by providing them as separate arguments. Here’s an example:
var element = document.getElementById("myElement");
element.classList.add("newClass1", "newClass2", "newClass3");
In this code snippet, we retrieve the element with the id “myElement” using document.getElementById and then use the .classList.add() method to add three new classes (newClass1, newClass2, newClass3) to the element.
- setAttribute(): When using the setAttribute() method to add multiple classes, the class names should be provided as a space-separated string. Here’s an example:
var element = document.getElementById("myElement");
element.setAttribute("class", "newClass1 newClass2 newClass3");
In this code snippet, we retrieve the element with the id “myElement” using document.getElementById and then use the setAttribute() method to set the class attribute to “newClass1 newClass2 newClass3”, where the classes are separated by spaces.
Both methods achieve the same result of adding multiple classes to an element. However, it’s important to note that when using setAttribute() to add multiple classes, it will replace any existing classes on the element with the new classes specified in the string.
Adding a Class Conditionally
JavaScript allows you to conditionally add a class to an HTML element based on specific conditions. Let’s explore how this can be achieved using the .classList.contains(), .classList.remove(), and .classList.add() methods:
var element = document.getElementById("myElement");
if (element.classList.contains('oldClass')) {
element.classList.remove('oldClass');
element.classList.add('newClass');
}
In the above code snippet, we first retrieve the element with the id “myElement” using document.getElementById. We then use the .classList.contains() method to check if the element has the class “oldClass”. If the condition evaluates to true, we proceed to remove the “oldClass” using the .classList.remove() method. Finally, we add the “newClass” using the .classList.add() method.
This approach allows for conditional class manipulation. If the element initially has the “oldClass”, it will be removed and replaced with the “newClass”. However, if the element does not have the “oldClass”, no changes will be made.
Adding a Class on Event
You can add a class to an HTML element based on an event, such as a button click or a mouse hover. JavaScript provides a convenient way to achieve this. Let’s take an example of adding a class on a button click:
var button = document.getElementById("myButton");
var element = document.getElementById("myElement");
button.onclick = function() {
element.classList.add('newClass');
}
In the above code snippet, we first retrieve the button element with the id “myButton” and the target element with the id “myElement” using document.getElementById. We then assign a function to the onclick event of the button using the button.onclick syntax. Inside the function, we use the .classList.add() method to add the class “newClass” to the target element.
When the button is clicked, the assigned function will be triggered, and the “newClass” will be added to the target element. This allows you to apply different styles or behavior to the element dynamically in response to user actions.
Conclusion
The power of JavaScript in altering web page content and styles is immense. Adding classes to HTML elements is just one feature of this versatile language, but it’s a fundamental one that every web developer should master.
FAQ
Absolutely! JavaScript allows you to select multiple elements using document.querySelectorAll() and iterate over them with a loop to add a class to each one.
JavaScript gracefully handles this by simply ignoring the command. The class will not be added again, and no error will be thrown.
JavaScript provides various event handlers, such as onmouseover for hovering and onfocus for focusing, which can be used in combination with class addition methods.
To add multiple classes with .className, you can provide a string with the class names separated by spaces. But remember, .className will replace any existing classes on the element.
The classList property provides a .remove() method, which can be used to remove a class from an element. You simply call element.classList.remove(‘className’).