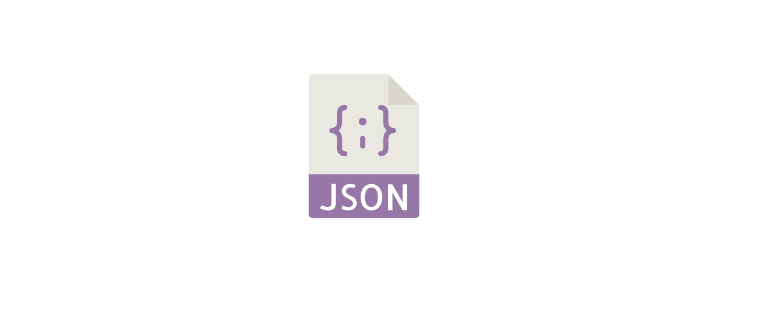
JSON (JavaScript Object Notation) is a lightweight data interchange format that’s easy to read and write for humans and easy to parse and generate for machines. It’s a text format that is completely language independent but uses conventions familiar to programmers of the C family of languages, which includes C, C++, C#, Java, JavaScript, Perl, Python, and a multitude of others.
JavaScript, as a powerful and widely-used programming language, has built-in functions to convert from JSON data format to JavaScript objects, and vice versa. In this article, we delve into the specifics of how to read JSON files using JavaScript.
Reading JSON Files in JavaScript: Node.js Perspective
In JSON, data is structured through key-value pairs, much like a JavaScript object. Arrays are represented by square brackets [] and objects by curly braces {}. This simple and intuitive format makes JSON a favorite for data storage and transmission.
Here is a simple example of JSON data:
{
"name": "John Doe",
"age": 30,
"city": "New York"
}
Node.js and the File System (fs) Module
Node.js is a versatile platform that allows the execution of JavaScript code outside of a web browser environment. It offers a range of modules, including ‘http’, ‘url’, ‘querystring’, ‘fs’, and more. These modules provide functions that enable Node.js to interact with the underlying operating system.
One of these modules is the ‘fs’ module, which facilitates operations with the file system on a computer. The ‘fs’ module provides numerous functionalities for file manipulation, such as reading, creating, updating, and deleting files, as well as renaming files. In this article, we will specifically focus on reading JSON files using the ‘fs’ module.
The ‘fs’ module in Node.js includes methods that make it straightforward to read the contents of a JSON file. The basic steps to read a JSON file using the ‘fs’ module are as follows:
- Import the ‘fs’ module: Before using the ‘fs’ module, it needs to be imported into your Node.js application. You can import it using the following line of code:
const fs = require('fs');
- Read the JSON file: Once the ‘fs’ module is imported, you can use its functions to read the contents of a JSON file. The most commonly used method for reading files is fs.readFile(). This method takes the file path and an optional encoding parameter as arguments. Here’s an example:
fs.readFile('path/to/file.json', 'utf8', (err, data) => {
if (err) {
console.error(err);
return;
}
console.log(data);
});
- Parse the JSON data: The fs.readFile() method returns the contents of the JSON file as a string. To work with the data as JSON objects, you need to parse it using the JSON.parse() method. This will convert the JSON string into a JavaScript object. Here’s an example:
fs.readFile('path/to/file.json', 'utf8', (err, data) => {
if (err) {
console.error(err);
return;
}
const jsonData = JSON.parse(data);
console.log(jsonData);
});
By following these steps, you can easily read the contents of a JSON file using the ‘fs’ module in Node.js. Once the JSON data is parsed, you can manipulate it, access specific properties, or perform any other operations as required.
Reading a JSON File Using Node.js
To read a JSON file using Node.js, you can use the fs module, which provides functionality for interacting with the file system. Below is a detailed breakdown of the script that demonstrates how to read a JSON file:
var fs = require('fs');
In the first line, we import the fs module using the require function. This module is a built-in module in Node.js and does not require any external installation.
fs.readFile('test.json', 'utf8', function (err, data) { if (err) throw err; let json = JSON.parse(data); console.log(json); });
The readFile function is used to read the contents of a file. It takes three parameters: the file path, the encoding (optional), and a callback function. In this script, we pass ‘test.json’ as the file path and ‘utf8’ as the encoding, which specifies that the file should be read as a text file in UTF-8 format.
The callback function is executed when the file reading operation is complete. It takes two parameters: err and data. If an error occurs during the file reading process, the callback function throws an error by using the throw statement.
Assuming the file reading operation is successful, the contents of the file are available in the data parameter. We use JSON.parse() to parse the JSON data into a JavaScript object. The parsed object is stored in the json variable.
Finally, we log the parsed JSON object to the console using console.log(json).
Table: Script Explanation
Line | Code | Explanation |
---|---|---|
1 | var fs = require(‘fs’); | Import the fs module |
3 | fs.readFile(‘test.json’, ‘utf8’, | Read the contents of the file test.json using the readFile |
function (err, data) { | function | |
4 | if (err) throw err; | Throw an error if there is an error in the file reading operation |
5 | let json = JSON.parse(data); | Parse the JSON data into a JavaScript object |
6 | console.log(json); | Log the parsed JSON object to the console |
Understanding the Code
The script provided is aimed at reading a JSON file and displaying its contents as a JavaScript object. Let’s break down the code into smaller parts and understand what each section does.
- Importing the fs Module: The script begins by importing the fs module using the require function. The fs module allows interaction with the file system, providing the necessary functions to read and write files.
const fs = require('fs');
- Reading the JSON File: The fs.readFile() function is called next, which is responsible for reading the JSON file. It takes three arguments:
Aspect | Description |
---|---|
File name | In this case, the file name is ‘test.json’, but you can replace it with the name of your own JSON file. |
File encoding | JSON files are typically encoded in UTF-8, a widely used character encoding format. The argument ‘utf8’ specifies the file encoding. |
Callback function | This function is executed after the file is read. |
fs.readFile('test.json', 'utf8', (err, data) => { // Callback function code goes here });
- Callback Function: The callback function provided as the third argument in fs.readFile() is executed after the file is read. It takes two arguments: err and data.
Parameter | Description |
---|---|
err | The err parameter is an error object that will contain information about any errors that occurred during the file reading operation. If an error occurred, err will be truthy (i.e., it will have a non-null value). |
data | The data parameter will contain the text content of the file if it was read successfully. |
fs.readFile('test.json', 'utf8', (err, data) => { if (err) { throw err; } // Code to parse and display the JSON data goes here });
- Error Handling: Inside the callback function, the code first checks if an error occurred during the file reading operation. If err is truthy (i.e., it contains a non-null value), an error occurred, and the code throws an error using throw err;. This stops the execution of the code.
if (err) { throw err; }
- Parsing the JSON Data: If no error occurred during the file reading operation, the code proceeds to parse the data from the JSON file using JSON.parse(data). This function takes a JSON string as an argument and transforms it into a JavaScript object. The resulting object is stored in the json variable.
const json = JSON.parse(data);
- Displaying the Result: Finally, the code logs the resulting JavaScript object, json, to the console using console.log(json). If the file was read and parsed successfully, you will see the contents of the JSON file printed as a JavaScript object in your console.
console.log(json);
Reading JSON Files in JavaScript: Web Browser Perspective
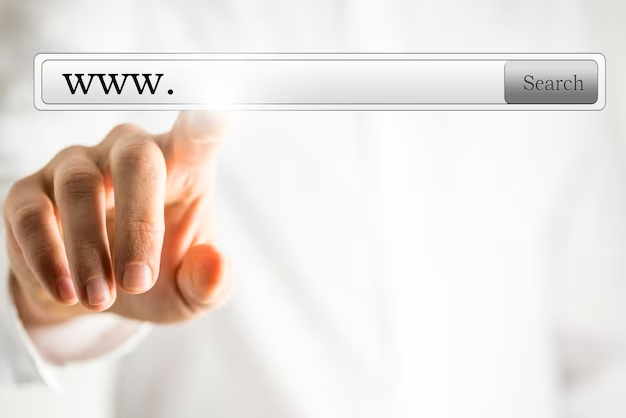
When running JavaScript in the web browser, accessing the file system directly is not allowed due to security reasons. However, we can retrieve JSON files from a server using the Fetch API. Here’s a simple example of how to fetch a JSON file in JavaScript:
fetch('test.json')
.then(response => response.json())
.then(data => console.log(data))
.catch((error) => console.log('Error:', error));
Let’s break down the code and explain each step:
Step | Description |
---|---|
Fetch | The fetch function is a built-in JavaScript function that allows us to make HTTP requests. In this case, we use it to fetch the test.json file from the server. |
Promise Chain | The fetch function returns a Promise that resolves to a Response object representing the HTTP response. We can chain methods like .then() and .catch() to handle the response. |
Response.json() | The first .then() method receives the response object and calls the json() method on it. This method returns another Promise that resolves to the actual JSON data in the response. |
Logging the Data | The second .then() method receives the JSON data as a parameter. In this example, we simply log the data to the console using console.log(). You can perform any desired operations on the data at this point. |
Error Handling | The .catch() method is used to handle any errors that may occur during the fetch or JSON parsing process. In this example, if an error occurs, we log an error message to the console. |
It’s important to note that the fetch operation is asynchronous, meaning it doesn’t block the execution of the rest of your JavaScript code. Instead, the Promise-based approach allows us to handle the response and data when they become available.
Understanding the Code
In the code snippet above:
- We call the fetch() function with the URL of the JSON file;
- We then chain a then() to the fetch() promise, where we call response.json(). This method reads the response body to completion and returns a promise that resolves with the result of parsing the body text as JSON;
- We chain another then() to log the data to the console;
- We add a catch() at the end to handle any errors that might occur during the fetch or parsing process.
Conclusion
Reading JSON files in JavaScript can be performed differently based on your environment: Node.js or a web browser. Understanding how to handle JSON data is a fundamental skill in JavaScript programming, due to the ubiquity of JSON as a data format on the web.
FAQ
Browsers restrict access to the local file system for security reasons. So, JavaScript running in a browser can’t directly read local files unless those files are selected by a user (e.g., via an input element of type ‘file’). However, if you’re running a local server, you can fetch JSON files from the local server as demonstrated above.
JSON.parse() is used to convert a JSON string into a JavaScript object. On the other hand, JSON.stringify() is used to convert a JavaScript object into a JSON string.
Yes, you can use fs.readFileSync() in Node.js. However, use this with caution as it blocks the execution of your code until the file is read, which can decrease performance if the file is large or if you’re reading multiple files.
The Fetch API provides an interface for fetching resources (including across the network). It’s more powerful and flexible than XMLHttpRequest, providing a more powerful and flexible feature set. It returns a Promise that resolves to the Response to that request, whether it is successful or not.
‘utf8’ is one of the character encodings supported in Node.js for reading text data. By specifying ‘utf8’, you’re telling Node.js to read the file content as a UTF-8 encoded text.
Yes, but it’s not built into Node.js like it is in browsers. You would need to install a package like node-fetch or axios to get similar functionality in Node.js