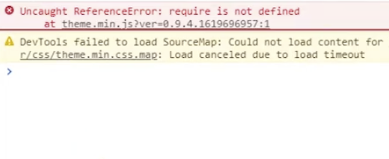
Error messages can be both a coder’s worst enemy and best friend. They are frustrating but also provide clues to problems in the code. One such enigmatic error that often baffles JavaScript developers is the “ReferenceError: require is not defined.” This article will serve as your guide to understanding, diagnosing, and resolving this issue with remarkable clarity.
Unmasking the Culprit: Why Does “Require is Not Defined” Occur?
Before we get into the nitty-gritty of solving the problem, it’s essential to understand why this error occurs. Knowing the root cause can make fixing the issue significantly more straightforward. So, why do you see “ReferenceError: require is not defined in JavaScript”?
Context | Description |
Context of Web Browsers | Nature of the Beast: The require method is a staple in Node.js for importing modules, but it’s not native to web browsers. This key difference makes the “js require is not defined” error pop up if you’re trying to use require in client-side JavaScript. |
Context of Non-Node.js Environments | The Non-Belonger: Since the required function is confined to the Node.js ecosystem, it becomes alien to other JavaScript runtime environments. Consequently, “require is not defined node js” isn’t applicable here; it’s more accurate to say “require is not defined in a non-Node.js environment.” |
Contextual Variations | Running Node.js but Still Failing: Sometimes, even in a Node.js context, due to incorrect configurations or folder structures, you might encounter the “node js require is not defined” error. |
Real-world Examples: When “Require is Not Defined” Strikes
Understanding the nuances of JavaScript environments can be challenging, especially when your code returns errors like “require is not defined” or “js module is not defined”. These issues typically manifest when there is a mismatch between the module system you’re using and the JavaScript environment where your code is running. Let’s delve into why these errors occur and how to resolve them.
Node.js Environment vs. Browser Environment
Property | Node.js | Browser |
Module System | CommonJS | ECMAScript Modules |
Syntax | require | import/export |
Context | Server-side | Client-side |
- Node.js: It employs CommonJS for module management. Using require to import modules is standard practice.
- Browser: Natively, modern browsers use ECMAScript Modules (ESM), which use import/export syntax for module management.
Why “Require is Not Defined” Occurs
In a browser environment, the required function is not defined because browsers don’t natively support CommonJS modules. As a result, attempting to use require throws the error.
Overcoming the “JS Require is Not Defined” error enhances JavaScript projects, indirectly boosting SEO and link-building efforts. Learn how this connects to optimizing link-building KPIs.
Why “JS Module is Not Defined” Occurs
The “js module is not defined” error can occur for similar reasons, but it is more generic. It often appears when the module or package you’re trying to import hasn’t been properly included in your project, or when there’s a syntax error.
Roll Up Your Sleeves: Fixing the Issue
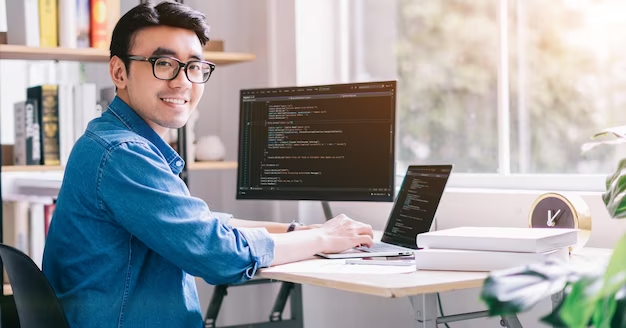
When you encounter the “js require is not defined” error, it can be frustrating, but the silver lining is that this problem is commonly faced and has known solutions. The “js require is not defined” error generally arises in different contexts, and your approach to resolving it should vary based on those specifics.
The Classic <script> Tag Method
<script src=”your-script.js”></script> |
Table: Advantages and Disadvantages
Aspect | Advantage | Disadvantage |
Simplicity | Very straightforward to implement. | May not handle complex dependencies. |
Synchronous | Loads files in the order they appear. | Blocks the rendering of other elements on the page. |
If you see the “js require is not defined” error when you’re trying to use this method, ensure that the script file actually exists and is accessible at the specified path.
Module Loaders to the Rescue
Example Syntax Using RequireJS:
require([‘some-dependency’], function(someDependency) { // Your code here }); |
Table: Advantages and Disadvantages
Aspect | Advantage | Disadvantage |
Dependencies | Manages complex dependencies efficiently. | Requires configuration. |
Asynchronous | Non-blocking; improves page load performance. | Slightly steeper learning curve. |
If you’re still facing the “js require is not defined” error, make sure that your configuration file for the module loader is set up correctly.
When the “js require is not defined” Error Occurs in a Non-Node.js Environment
Environment-Specific Loader
- Python: Use import statements.
- C#: Use using directives.
Global Objects
- Adobe’s ExtendScript: Use #include for importing scripts.
#include “someScript.jsx” |
If the “js require is not defined” error appears in these environments, consult the specific language or environment documentation for module or script inclusion.
When the “js require is not defined” Error Occurs in Node.js but You’re Still Facing Issues
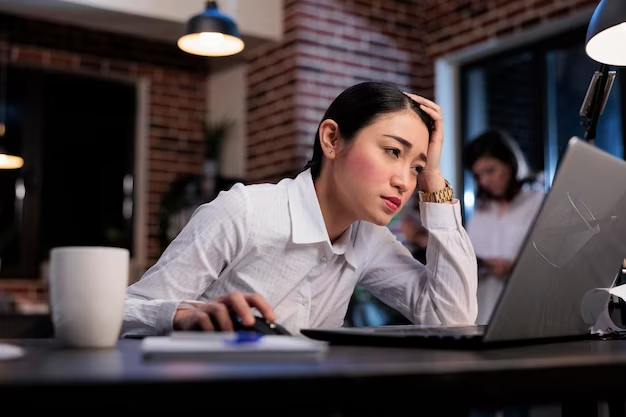
Check Your Paths
- Relative path example: const myModule = require(‘./myModule’);
- Absolute path example: const myModule = require(‘/full/path/to/myModule’);
When the “js require is not defined” error persists, double-check that your paths are correctly defined.
Inspect Node Modules
- Run: npm install
- Check: package.json for dependencies
Even after running the install, if you see the “js require is not defined” error, validate that the required packages are listed in package.json.
Examine Code Structure for Circular Dependencies
Example of Circular Dependency:
- File A requires File B
- File B requires File C
- File C requires File A
How to Resolve:
- Refactor your code to break the loop.
- Use lazy-loading techniques to defer the required call.
If you’ve done all this and still face the “js require is not defined” issue, consider restructuring your code to avoid circular dependencies.
A Stitch in Time: Proactive Error Management with Tools
Errors and bugs are an inevitable part of software development. One common error that developers encounter is the dreaded “ReferenceError: require is not defined” in JavaScript. In this article, we will explore this error, its causes, and most importantly, proactive error management tools and practices to help you save time and streamline your development process.
Proactive Error Management
Dealing with errors reactively can be time-consuming and frustrating. Proactive error management involves taking steps to prevent errors from occurring in the first place and having tools and practices in place to catch and address them when they do happen.
Code Linting
Code linting is a static analysis of your code to identify issues before runtime. Tools like ESLint for JavaScript and TSLint for TypeScript can help you catch potential problems, including issues related to the usage of require. They can enforce coding standards, highlight syntax errors, and ensure code consistency.
Unit Testing
Unit testing involves writing test cases for individual units (functions, modules, or classes) of your code. Frameworks like Jest and Mocha provide powerful tools for writing and running tests. By creating test cases that cover different scenarios, you can detect and fix errors, including “require is not defined,” early in the development process.
Dependency Management
Properly managing your project’s dependencies is crucial. Use package managers like npm or Yarn to ensure that all required modules are installed correctly and that their versions are compatible with your project.
Error Tracking and Monitoring
Automated error-tracking software plays a significant role in proactive error management. Tools like Rollbar continuously monitor your code in real-time. They capture and log errors, including “ReferenceError: require is not defined,” along with valuable information like stack traces and user context. This enables you to:
- Receive instant notifications when an error occurs.
- Analyze error patterns and trends.
- Prioritize and fix critical issues quickly.
- Improve user experience by addressing issues before they impact users.
Code Review and Collaboration
Collaboration with your team can help identify and prevent errors. Regular code reviews allow team members to catch issues like missing require statements or incorrect file paths early in the development process.
Using Rollbar for Proactive Error Management
Rollbar is a robust error tracking and monitoring tool that can significantly enhance your proactive error management efforts. Here’s how it can help with the “ReferenceError: require is not defined” error:
- Real-Time Error Notifications: Rollbar instantly notifies you when this error occurs, allowing you to address it promptly.
- Detailed Error Reports: It provides detailed error reports, including the stack trace, environment information, and user context, making debugging easier.
- Error Trend Analysis: Rollbar helps you identify trends in errors, allowing you to proactively address issues that are affecting your application.
- Integration with Build and Deployment Tools: You can integrate Rollbar into your CI/CD pipeline to catch errors before they reach production.
By incorporating Rollbar into your workflow, you can ensure that errors like “ReferenceError: require is not defined” are detected and resolved efficiently, saving you time and improving the quality of your code.
Conclusion
Debugging is a fundamental skill in coding, and errors like “js require is not defined” are your playground for mastering it. Hopefully, this article has demystified this common JavaScript error for you. You’re now equipped with the knowledge and solutions to fix “js require is not defined” wherever it occurs. And remember, whenever you’re stuck with this error, you now know what steps to take to debug and fix it efficiently.
FAQs
Why do I face “js require is not defined” when coding in a browser?
require is specific to Node.js and not native to web browsers. This mismatch results in the error.
Can I use ES6 imports in Node.js as an alternative?
Yes, you can, but ensure your project configuration allows ES6 imports.
How can I fix “require is not defined” in a non-Node.js environment?
Use the methods or objects provided by that specific environment to load external modules.
Why does “node js require is not defined” happen even in a Node.js environment?
Incorrect file paths, missing Node modules, or circular dependencies can sometimes cause this error even in a Node.js context.