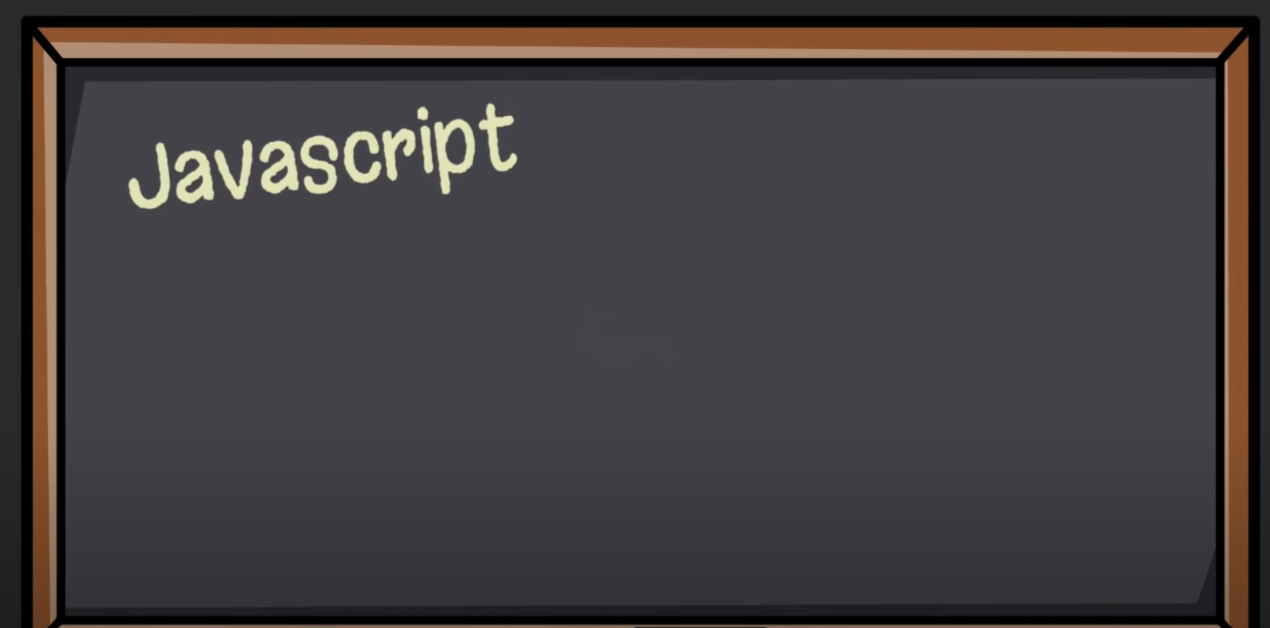
Understanding JavaScript’s capabilities for number formatting is vital for any developer, as it can significantly enhance the clarity of numerical data in your application. This comprehensive article presents several methods of formatting numbers in JavaScript, covering built-in methods, international formatting, and third-party libraries.
JavaScript Number Format Fundamentals
In JavaScript, the Number object is the basis of all numerical data handling. This object can represent integers and floating-point numbers. JavaScript provides several built-in methods for interacting with these objects, but it doesn’t offer inherent ways to format numbers. As a developer, you need to create or use existing solutions for adding thousands separators, leading zeros, and limiting decimal places, amongst other tasks.
let num = new Number(123456.789);
Adding Leading Zeros
In JavaScript, you often need to add leading zeros to single-digit numbers, especially when working with dates and times. For example, you may want to display single-digit months as ’01’, ’02’, ’03’, and so forth.
Here’s a simple technique that leverages string concatenation and slicing:
let month = 5;
let formattedMonth = ("0" + month).slice(-2);
console.log(formattedMonth); // outputs '05'
This code prepends a “0” to the number and then uses the slice(-2) function to extract the last two characters. The result is a uniformly two-digit number regardless of the initial value.
To extend this approach to more than two digits, use a loop that adds zeros until the string reaches the desired length.
Enforcing Decimal Places
In applications that manage financial data or scientific calculations, you often need to display numbers with a specific number of decimal places. JavaScript provides a built-in method for this task: toFixed(). This method formats a number with a specified number of digits after the decimal point.
let num = 123.456;
let formattedNum = num.toFixed(2);
console.log(formattedNum); // outputs '123.46'
In this example, toFixed(2) rounds the number to the nearest hundredth, so the output always includes exactly two digits after the decimal point.
Thousands Separator
Adding a thousands separator can significantly improve readability for large numbers. While JavaScript doesn’t provide a built-in method for this, you can achieve it with a regular expression:
let num = 123456789;
let formattedNum = num.toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",");
console.log(formattedNum); // outputs '123,456,789'
This code converts the number to a string and uses the replace() function with a regular expression to add commas as thousands separators.
Number Formatting with Locale Methods
toLocaleString()
JavaScript’s toLocaleString() method is a powerful tool for formatting numbers based on specific locales and formatting preferences. It simplifies tasks such as currency formatting and the inclusion of thousands separators. Let’s take a closer look at the usage and syntax of this method using an example:
let num = 123456.789;
console.log(num.toLocaleString('en-US', {style: 'currency', currency: 'USD'}));
// outputs '$123,456.79'
In the code snippet above, we apply the toLocaleString() method to the number 123456.789. By specifying the ‘en-US’ locale, the number is formatted according to the conventions used in the United States. The resulting formatted string includes a comma as a thousands separator and the dollar symbol, creating the output ‘$123,456.79’.
Intl.NumberFormat
When you need to perform repetitive number formatting operations, using the Intl.NumberFormat method in JavaScript offers improved efficiency compared to toLocaleString(). With Intl.NumberFormat, you can create a reusable formatter that enhances the performance of your application. Let’s explore an example:
let num = 123456.789;
let formatter = new Intl.NumberFormat('en-US', {style: 'currency', currency: 'USD'});
console.log(formatter.format(num)); // outputs '$123,456.79'
In the above code snippet, we initialize a formatter using Intl.NumberFormat with the ‘en-US’ locale and the desired formatting options. The resulting formatter object can be used multiple times with different numbers, avoiding the need to redefine the formatting rules each time. In this case, formatter.format(num) formats the number 123456.789 as a currency value in US dollars, producing the output ‘$123,456.79’.
Formatting Numbers with Libraries
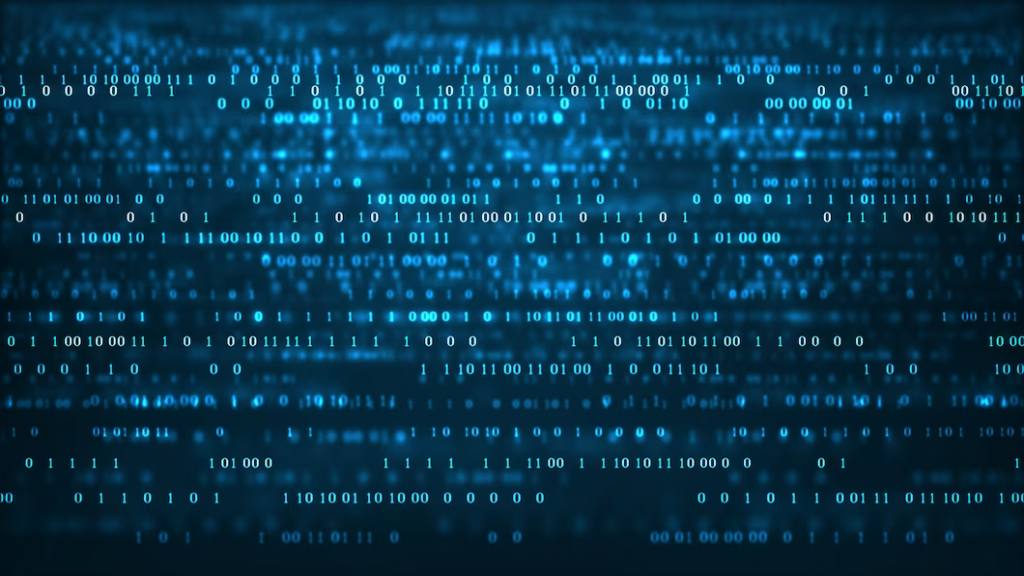
Formatting numbers in JavaScript can be accomplished using various third-party libraries that offer specialized and powerful tools. Here, we will explore three popular libraries: Numeral.js, accounting.js, and Intl.js. These libraries provide different formatting options and can be included in your project through CDN links or package managers like npm.
Numeral.js
Numeral.js is a versatile library that offers a wide range of formatting options for numbers. Some of the formats supported by Numeral.js include currency, percentage, time, ordinal, and decimal formats. To use Numeral.js, you can include the library in your project by adding a CDN link or installing it via npm.
Here’s an example of how Numeral.js can be used to format a number as currency:
const number = 12345.6789;
const formattedNumber = numeral(number).format('$0,0.00');
console.log(formattedNumber); // Output: $12,345.68
accounting.js
accounting.js is a library specifically designed for financial calculations and formats. It provides features like currency formatting and accounting notation. You can include accounting.js in your project by adding a CDN link or installing it via npm.
Here’s an example of how accounting.js can be used to format a number as currency:
const number = 12345.6789;
const formattedNumber = accounting.formatMoney(number, '$', 2);
console.log(formattedNumber); // Output: $12,345.68
Intl.js
Intl.js is a library that aims to provide an ECMAScript-compatible internationalization solution when the native API is unavailable or insufficient. It provides various formatting options for numbers, dates, and currencies. To use Intl.js, you can include the library in your project by adding a CDN link or installing it via npm.
Here’s an example of how Intl.js can be used to format a number as currency:
const number = 12345.6789;
const formattedNumber = new Intl.NumberFormat('en-US', { style: 'currency', currency: 'USD' }).format(number);
console.log(formattedNumber); // Output: $12,345.68
Remember to include the necessary script tags or import statements in your HTML or JavaScript file when using these libraries. By leveraging the capabilities of these libraries, you can easily format numbers according to your specific requirements in JavaScript projects.
Deep Dive into Numeral.js
Numeral.js is a popular library due to its versatility. To use it, include the library via a script tag in your HTML file or install it using npm and import it into your JavaScript file.
<script src="path/to/numeral.min.js"></script>
Once included, you can use the numeral() function to create a Numeral object, then use the format() function to format your number:
let num = 123456.789;
let formattedNum = numeral(num).format('0,0.00');
console.log(formattedNum); // outputs '123,456.79'
In the format() function, ‘0,0.00’ is a string that describes how the number should be formatted. Here, the comma represents the thousands separator, and ‘.00’ enforces two decimal places.
Numeral.js supports a plethora of format strings, providing flexibility for various scenarios:
- ‘0,0’: No decimal places, with a comma as the thousands separator;
- ‘0.0’: One decimal place, no thousands separator;
- ‘0.00’: Two decimal places, no thousands separator;
- ‘$0,0.00’: Two decimal places with a comma as the thousands separator and a dollar sign for currency.
Conclusion
JavaScript number formatting is a crucial skill for any web developer. While JavaScript doesn’t natively support all types of number formatting, there are ample techniques and resources available to handle any formatting need. Whether you’re adding leading zeros, limiting decimal places, adding thousands separators, or implementing international formatting, you now have a range of solutions at your fingertips. With these tools in hand, you can make numerical data in your applications clear, consistent, and user-friendly.
FAQ
The best way depends on the specific formatting task. For simple operations like adding leading zeros or specifying decimal places, built-in JavaScript methods like slice() or toFixed() are efficient and easy to use. For more complex tasks like international formatting or repeated formatting operations, toLocaleString() and Intl.NumberFormat are excellent tools. If you require powerful formatting capabilities or need to format numbers consistently across a large application, consider using a library like Numeral.js.
Use the toFixed() method:
let num = 5.6789;
let formattedNum = num.toFixed(2);
console.log(formattedNum); // outputs ‘5.68’
The toFixed() method can format decimal numbers by specifying the number of digits after the decimal point:
let num = 5.6789;
let formattedNum = num.toFixed(3);
console.log(formattedNum); // outputs ‘5.679’
This code will output the number rounded to the nearest thousandth.
A: Use the toLocaleString() method for one-off operations:
let num = 1234567.89;
let formattedNum = num.toLocaleString(‘en-US’);
console.log(formattedNum); // outputs ‘1,234,567.89’
Or use Intl.NumberFormat for repeated operations:
let num = 1234567.89;
let formatter = new Intl.NumberFormat(‘en-US’);
console.log(formatter.format(num)); // outputs ‘1,234,567.89’
In both examples, ‘en-US’ is the locale, which, among other things, determines the thousands separator.