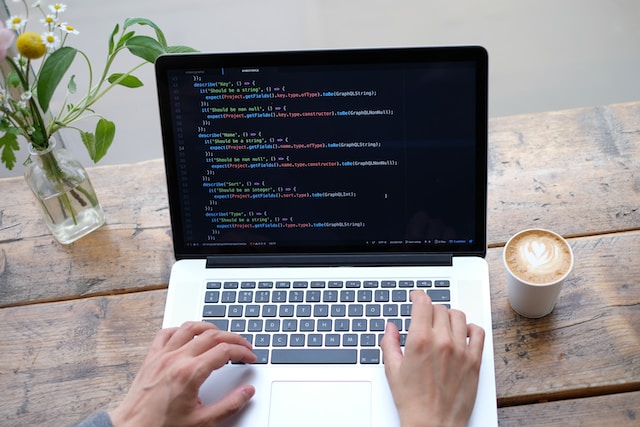
JavaScript is a versatile and powerful programming language that has revolutionized the way websites and web applications are created. One of the fundamental features of JavaScript is the ability to manipulate the DOM, which stands for Document Object Model.
By using JavaScript, web developers can create dynamic and interactive web pages that can respond to user input and update content on the fly. The AppendChild method is one such feature that is commonly used to manipulate the DOM.
The Basics of AppendChild in JavaScript
At its core, the AppendChild method is used to add a child element to a parent element in the DOM. This can be useful when you need to dynamically add content to a web page or modify existing content.
Appending child elements to a parent element can be done using various methods in JavaScript. However, the AppendChild method is the most commonly used method for this purpose.
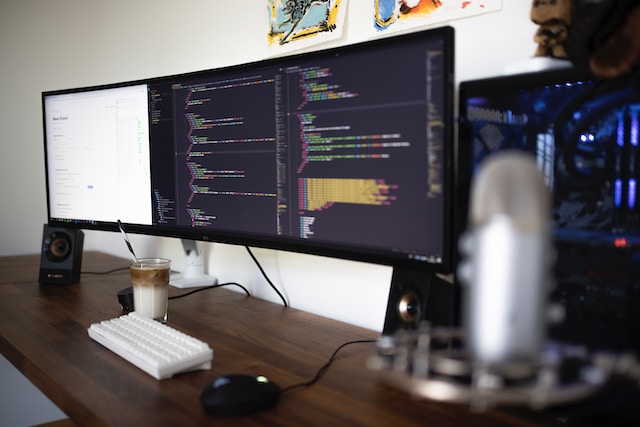
What is AppendChild?
The AppendChild method is a DOM method that is used to add a new child node to an existing parent node. This means that you can add new elements to an existing HTML document using JavaScript.
The AppendChild method is not limited to adding only HTML elements as child nodes. You can also add text nodes, comments, and other types of nodes as child nodes using this method.
The Syntax of AppendChild
The syntax for using the AppendChild method is straightforward. To add a new child node to an existing parent node, you need to use the following syntax:
parentElement.appendChild(childElement);
Here, parentElement is the ID or class name of the parent element you want to append a child to, and childElement is the element you want to add to the parent.
It is important to note that the AppendChild method only adds the child element to the end of the parent element’s list of children. If you want to add the child element at a specific position within the list of children, you will need to use a different method.
When to Use AppendChild
There are many situations where the AppendChild method can be useful. For instance, you might want to add new content to a web page based on user input or update existing content dynamically. You can also use the AppendChild method to create dynamic lists or tables that update based on user interaction.
Additionally, the AppendChild method can be used in conjunction with other DOM methods to create complex web applications. For example, you can use the AppendChild method to add new elements to a web page based on the results of an AJAX request.
Working with DOM Elements
Before we dig deeper into the AppendChild method, let’s understand how we can create and manipulate DOM elements in JavaScript.
The Document Object Model (DOM) is a programming interface for web documents. It represents the page so that programs can change the document structure, style, and content.
JavaScript is used to interact with the DOM and create dynamic HTML pages.
Creating New DOM Elements
To create a new DOM element using JavaScript, you can use the document.createElement() method. This method takes a single argument, which is the HTML tag of the element you want to create.
For instance, if you want to create a new div element, you can use the following code:
var newDiv = document.createElement(‘div’);
You can then add attributes to the new element using the setAttribute() method.
For example, to set the class of the new div element to ‘myClass’, you can use the following code:
newDiv.setAttribute(‘class’, ‘myClass’);
Accessing Existing DOM Elements
You can also access existing DOM elements using JavaScript. To do this, you can use methods like getElementById or getElementsByClassName.
For instance, if you want to access an element with the ID ‘myDiv’, you can use the following code:
var myDiv = document.getElementById(‘myDiv’);
You can then modify the existing element using various methods.
Modifying DOM Elements
Once you have created or accessed a DOM element using JavaScript, you can modify it using various methods. For example, you can change the text content of an element using the innerHTML property.
For instance, if you want to change the text content of an element with the ID ‘myDiv’, you can use the following code:
document.getElementById(‘myDiv’).innerHTML = ‘New text content’;
You can also change the attributes of an element using the setAttribute() method.
For example, to change the class of an element with the ID ‘myDiv’ to ‘newClass’, you can use the following code:
document.getElementById(‘myDiv’).setAttribute(‘class’, ‘newClass’);
Overall, working with DOM elements in JavaScript is essential for creating dynamic and interactive web pages.
Practical Examples of AppendChild
Now that we understand the basics of the AppendChild method and working with DOM elements, let’s look at some practical examples of how to use AppendChild in JavaScript.
Appending child elements to a parent element is a powerful technique that can help us build dynamic web pages and applications. Here are some more examples of how we can use the AppendChild method to create dynamic content.
Adding a New Paragraph to an Existing Div Element
The AppendChild method can be used to add a new paragraph to an existing div element. This can be useful when we want to add additional content to a web page without reloading the entire page. Here’s an example:
var myDiv = document.getElementById(‘myDiv’);var newParagraph = document.createElement(‘p’);newParagraph.innerHTML = ‘This is a new paragraph.’;myDiv.appendChild(newParagraph);
Creating a Dynamic Image Gallery
The AppendChild method can also be used to create a dynamic image gallery that updates based on user input. For instance, if you have an empty div element and want to add images to it dynamically, you can use the following code:
var imageGallery = document.getElementById(‘imageGallery’);var newImage = document.createElement(‘img’);newImage.src = ‘image.jpg’;imageGallery.appendChild(newImage);
This will add a new image to the image gallery every time the user uploads a new image.
Building a Dynamic Navigation Menu
The AppendChild method can also be used to create a dynamic navigation menu that updates based on the user’s actions. For instance, if you have an empty unordered list and want to add new menu items to it dynamically, you can use the following code:
var navMenu = document.getElementById(‘navMenu’);var newMenuItem = document.createElement(‘li’);newMenuItem.innerHTML = ‘New menu item’;navMenu.appendChild(newMenuItem);
This will add a new item to the navigation menu every time the user clicks on a link or button.
Adding a New Section to an Existing Web Page
The AppendChild method can also be used to add a new section to an existing web page. For instance, if you have an existing web page and want to add a new section to it dynamically, you can use the following code:
var body = document.getElementsByTagName(‘body’)[0];var newSection = document.createElement(‘section’);newSection.innerHTML = ‘
New section
This is a new section.
‘;body.appendChild(newSection);
This will add a new section to the web page every time the user clicks on a link or button.
Common Issues and Solutions with AppendChild
Although the AppendChild method is a powerful tool for manipulating the DOM, it can also be the source of various issues if not used correctly.
Dealing with Duplicate Elements
One common issue with the AppendChild method is duplicate elements. If you try to add a child element to a parent element that already contains the same child, you might end up with duplicate elements.
To avoid this issue, you can check if the child element already exists in the parent before appending it using the following code:
if (!parentElement.contains(childElement)) { parentElement.appendChild(childElement);}
Handling Cross-Browser Compatibility
Another issue with the AppendChild method is cross-browser compatibility. Different browsers might interpret the AppendChild method slightly differently, leading to unexpected behavior.
To avoid this issue, you can use a library like jQuery, which abstracts away many of the cross-browser compatibility issues.
Troubleshooting Performance Issues
Finally, the AppendChild method can be a source of performance issues if used incorrectly. For instance, adding too many child elements to a parent can slow down the browser.
To avoid this, you should use the AppendChild method sparingly and consider alternative approaches like lazy loading or pagination for displaying large amounts of content.
Conclusion
The AppendChild method is a powerful feature of JavaScript that allows you to manipulate the DOM dynamically. By understanding how to use AppendChild, you can create dynamic and interactive web pages that respond to user input and update content on the fly. So if you are a web developer looking to create modern and engaging web applications, make sure to add the AppendChild method to your toolkit.