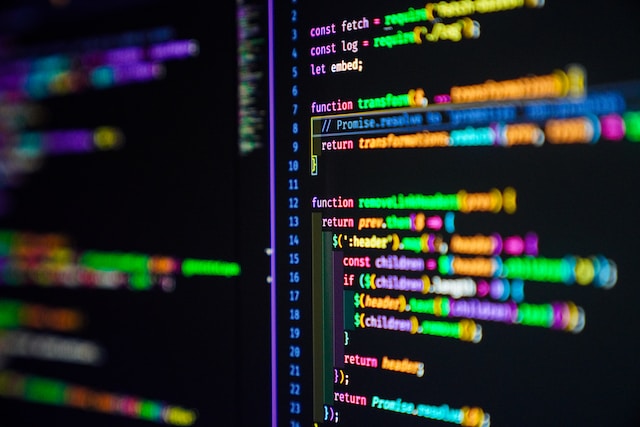
Developing a flexible and robust JavaScript function often requires dealing with optional arguments. Optional arguments are parameters that may or may not be passed to a function call. They add to a function’s flexibility and allow for more customization. However, handling multiple optional arguments can be a challenging task, which requires a sound understanding of the different approaches and best practices.
Understanding Optional Arguments in JavaScript
Optional arguments refer to parameters that a function may or may not receive during a function call. They do not have to be defined or specified in the function’s parameter list. When an optional argument is not provided, the function can either use default values or entirely skip the operation.
What are Optional Arguments?
Optional arguments are additional parameters that may or may not be passed to a function call. Unlike mandatory arguments, optional arguments do not require an input value or a default value.
Why Use Optional Arguments?
The primary reason for using optional arguments is to develop flexible and versatile functions. Optional arguments allow developers to customize a function call and perform more tasks while giving more control to the user.
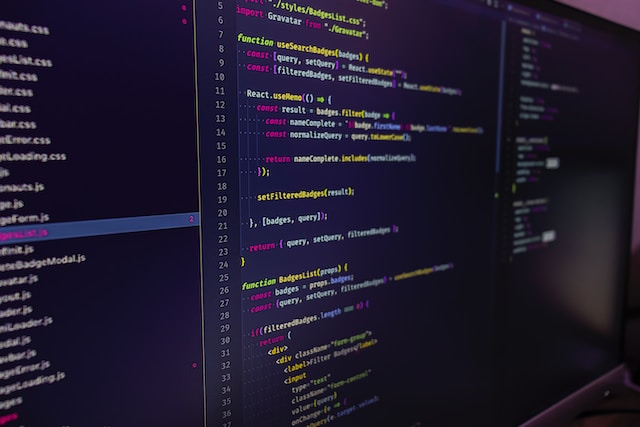
Approaches to Handling Optional Arguments
Optional arguments are parameters in a function that are not required to be passed in when the function is called. There are different approaches to handle optional arguments within a function. Some of the commonly used approaches include:
Using Default Parameter Values
Using default parameter values is a common approach to handle optional arguments. It involves defining a default value for the optional parameter within the function’s parameter list. If the argument is not provided, the default value is used. This approach is useful when the function has a small number of optional arguments, and the default values are simple.
For example, consider a function that calculates the area of a rectangle. The function can have optional arguments for length and width. If the arguments are not provided, the function can use default values of length = 1 and width = 1.
Utilizing the Arguments Object
The arguments object is an array-like object available within a function that allows developers to access all the function call’s arguments. The arguments object can be used to check if an argument was provided and set a default value. This approach is useful when the function has a large number of optional arguments, and the default values are complex.
For example, consider a function that calculates the total cost of a shopping cart. The function can have optional arguments for discounts, taxes, and shipping costs. If the arguments are not provided, the function can use default values based on the user’s location and the current date.
Implementing the Rest Parameter Syntax
The rest parameter syntax allows functions to accept an indefinite number of arguments as an array. The rest parameter is defined as a variable that captures all the remaining arguments in the function call. Developers can then loop over the array and perform appropriate tasks. This approach is useful when the function has a variable number of optional arguments.
For example, consider a function that calculates the average of a set of numbers. The function can have a variable number of arguments. If no arguments are provided, the function can return an error message. If one or more arguments are provided, the function can calculate the average.
In conclusion, handling optional arguments is an essential aspect of function design. Developers should choose an approach that suits the function’s requirements and complexity. Using default parameter values, utilizing the arguments object, and implementing the rest parameter syntax are some of the commonly used approaches.
Best Practices for Working with Optional Arguments
Optional arguments are a powerful tool for making a function flexible and versatile. However, they can also create readability and maintainability issues if not handled properly. Here are some best practices for working with optional arguments:
Keeping Code Readable and Maintainable
While optional arguments can be useful, defining too many of them can quickly make your code difficult to read and maintain. Instead, consider grouping optional arguments within an options object. This approach can help make your function’s purpose clear and make it easier to document. Additionally, it’s important to ensure that your function has a clear and descriptive name that accurately reflects its purpose.
Validating and Sanitizing Input
When working with optional arguments, it’s important to validate and sanitize input parameters to avoid unexpected errors or security threats. Input validation ensures that your function only receives the expected number of arguments of the correct type and format. Additionally, input sanitization helps to prevent malicious input from causing harm to your application or users.
Avoiding Common Pitfalls
There are several common pitfalls to watch out for when working with optional arguments. One of the most important is to ensure that your function handles optional arguments independently and unconditionally. This means that your function should not rely on the presence or absence of optional arguments to determine its behavior. Instead, it should always check for the presence of optional arguments and handle them appropriately.
Another important consideration is to use appropriate default values for optional arguments. This can help to ensure that your function behaves predictably even if optional arguments are not provided. Finally, avoid using the arguments object in an arrow function. The arguments object is not available in arrow functions, and attempting to use it can result in unexpected behavior.
By following these best practices, you can ensure that your functions are flexible, maintainable, and easy to use.
Real-World Examples of Multiple Optional Arguments
Let’s explore some real-world examples of how to handle multiple optional arguments.
Customizing a Data Fetching Function
A data fetching function that displays a list of products can have optional arguments such as the number of products to be displayed and their order. By grouping these optional arguments in an options object, developers can customize the function to meet different user needs.
Enhancing a Logging Utility
A logging utility function can have multiple optional arguments, such as message severity, log format, and output destination. These optional arguments can enhance the logging utility’s functionality by creating custom logs and providing more control to the user.
Building a Flexible Configuration Object
A configuration object with optional arguments can control various feature flags of an application. Adding an options object that contains optional arguments can enable users to turn specific features on or off, overriding the application defaults.
Conclusion
Optional arguments are a useful tool in developing a flexible and robust JavaScript function. Using appropriate approaches and best practices can help handle multiple optional arguments without creating readability or maintainability issues.