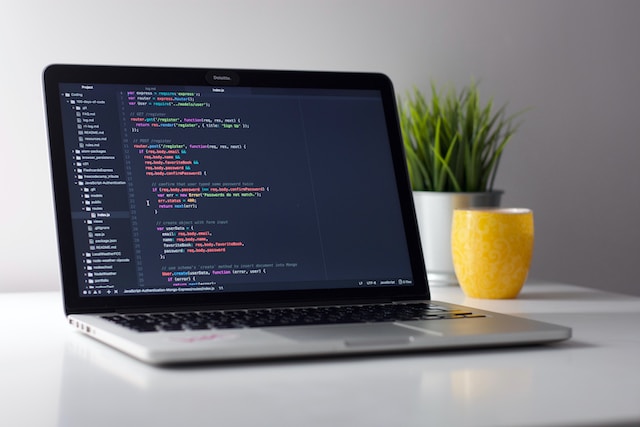
If you’re a JavaScript developer, you have likely heard of the Splice method. But do you truly understand what it does and how it works? In this article, we will explore the Splice method in depth, discussing its syntax, functionality, common use cases, and potential pitfalls. Whether you’re a beginner or an experienced developer, this article will provide valuable insights into this crucial JavaScript method.
Understanding the Splice Method in JavaScript
Before we dive into the details of Splice, let’s start with the basics. If you’ve worked with JavaScript, you know that they are mutable, meaning that their content can be modified after their creation. This feature makes one of the most powerful data structures in JavaScript. Arrays can store multiple values of different data types and allow you to access and manipulate them with ease.
One of the most powerful methods for modifying is the Splice method, which allows you to add, remove, and replace elements at specified positions. This method is widely used in JavaScript applications, and understanding how it works is essential for any developer who wants to work.
What is the Splice Method?
The Splice method is a built-in method in JavaScript that allows developers to change the contents by adding, removing, and replacing elements. By specifying the index at which to start the modification and the number of elements to modify, developers can make precise changes. This method is especially useful when you need to modify it without changing its length.
One of the most common use cases for the Splice method is removing elements from an array. If you want to remove one or more elements from an array, you can use the Splice method to do so. For example, if you have an array of numbers and you want to remove the first two elements, you can do so using the following code:
var numbers = [1, 2, 3, 4, 5];
numbers.splice(0, 2); // removes the first two elements
console.log(numbers); // [3, 4, 5]
In this example, we start the modification at index 0 and remove two elements from the array. The resulting array contains only the elements that were not removed.
Syntax of the Splice Method
The syntax of the Splice method is straightforward. It takes the following form:
array.splice(start[, deleteCount[, item1[, item2[, …]]]])
Let’s break down each part of this syntax:
- The array is the one that you want to modify. This is a required parameter;
- The start parameter is the index at which to begin making modifications. If negative, it specifies an offset from the end of the. This parameter is also required;
- The deleteCount parameter is optional and specifies the number of elements to remove from the array. If the parameter is not specified, all subsequent elements will be removed;
- The item1, item2, etc. parameters are optional and represent the elements to insert into the array at the specified start position. If you want to add elements to the array, you can include them as additional parameters.
The Splice method is a powerful tool for working with JavaScript. By understanding how it works and how to use it, you can write more efficient and effective code that manipulates with ease.
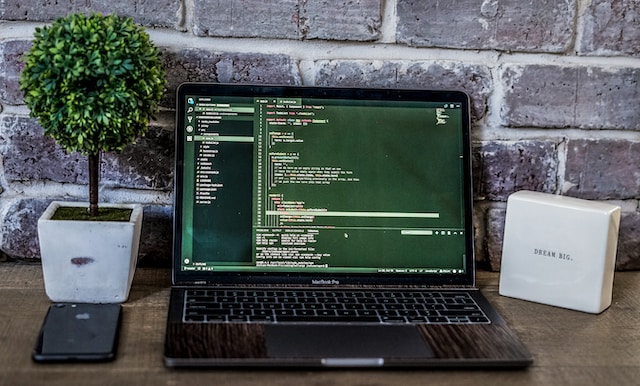
Alt: black laptop computer naer plant on brown wooden surface
How Splice Works: A Step-by-Step Guide
Now that you understand the basic syntax and functionality of the Splice method, let’s take a look at how it works in practice. First, we will explore how to add elements to and using Splice.
Adding elements to an is a common task in JavaScript programming. The Splice method provides an easy way to add one or more elements to an at a specified position. This can be useful when you need to insert new elements into an without overwriting existing elements.
Suppose that you have an of fruits as follows:
const fruits = [‘apple’, ‘banana’, ‘orange’, ‘peach’];
If we want to add ‘strawberry’ and ‘kiwi’ to the fruits after ‘banana’, we can use the Splice method as follows:
fruits.splice(2, 0, ‘strawberry’, ‘kiwi’);
Here’s how this code works:
- The first parameter, 2, tells Splice to start inserting elements at the third position (remember that JavaScript arrays are zero-indexed);
- The second parameter, 0, tells Splice that we want to insert elements, but not remove any elements;
- The third and fourth parameters, ‘strawberry’ and ‘kiwi’, respectively, are the elements we want to insert into the array.
If you log the fruits array to the console after running this code, you will see the following output:
[‘apple’, ‘banana’, ‘strawberry’, ‘kiwi’, ‘orange’, ‘peach’]
The Splice method has successfully added ‘strawberry’ and ‘kiwi’ to the fruits array at the second position.
Removing Elements from an Array
Another common task in JavaScript programming is removing elements from. The Splice method can be used to remove one or more elements from an at a specified position. This can be useful when you need to remove elements from an array without creating a new.
Suppose that we want to remove the ‘banana’ and ‘orange’ elements from the fruits array:
fruits.splice(1, 2);
Here’s how this code works:
- The first parameter, 1, tells Splice to start removing elements at the second position;
- The second parameter, 2, tells Splice to remove two elements (i.e., ‘banana’ and ‘orange’).
If you log the fruits to the console after running this code, you will see the following output:
[‘apple’, ‘peach’]
The Splice method has successfully removed the ‘banana’ and ‘orange’ elements from the fruits.
Replacing Elements in an Array
The Splice method can also be used to replace existing elements. Suppose that we want to replace the ‘orange’ element with a ‘mango’ element in the fruits array:
fruits.splice(2, 1, ‘mango’);
Here’s how this code works:
- The first parameter, 2, tells Splice to start replacing elements at the third position;
- The second parameter, 1, tells Splice to remove one element (i.e., ‘orange’);
- The third parameter, ‘mango’, is the element we want to insert into them at the specified position.
If you log the fruits to the console after running this code, you will see the following output:
[‘apple’, ‘banana’, ‘mango’, ‘peach’]
The Splice method has successfully replaced the ‘orange’ element with a ‘mango’ element in the fruits.
Practical Examples of Using Splice
Now that you understand how Splice works, let’s explore some practical use cases for the method in real-world JavaScript applications.
Manipulating Shopping Carts
Suppose that you are building an e-commerce website, and you want to allow customers to add and remove items from their shopping carts. You could use Splice to modify the cart based on the user’s actions. For example, to add an item to the cart at the specified index, you could use the following code:
cart.splice(index, 0, newItem);
To remove an item from the cart at the specified index, you could use the following code:
cart.splice(index, 1);
Using Splice in this way allows you to keep the contents of the shopping cart up-to-date and accurate based on the user’s actions.
Updating a To-Do List
Splice can also be useful for updating to-do lists in JavaScript applications. Suppose that you have an of tasks, and you want to allow users to mark tasks as completed and remove them from the list. You could use Splice to modify the tasks based on the user’s actions. For example, to mark a task as completed, you could use the following code:
tasks.splice(index, 1, updatedTask);
To remove a completed task from the tasks, you could use the following code:
tasks.splice(index, 1);
Using Splice in this way allows you to keep the to-do list up-to-date and organized based on the user’s actions.
Rearranging Items in a Playlist
Finally, Splice can be useful for rearranging items in a playlist. Suppose that you have any of the songs in a specific order, and you want to allow users to rearrange the songs by dragging and dropping them within the playlist. You could use Splice to modify the songs based on the user’s actions. For example, to move a song from one position to another within the, you could use the following code:
songs.splice(newIndex, 0, songs.splice(oldIndex, 1)[0]);
Using Splice in this way allows you to rearrange the order of songs in the playlist based on the user’s actions.
Common Mistakes and How to Avoid Them
While Splice is a powerful and versatile method, it is not without potential pitfalls. Here are some common mistakes that developers make when using Splice, and how to avoid them.
Confusing Splice with Slice
Splice and Slice are two different methods in JavaScript, but their names are similar and can be easily confused. Slice returns a new that contains a portion of the original, while Splice modifies the original. Be sure to double-check which method you are using to avoid unintended consequences.
Incorrectly Specifying Index and Delete Count
One of the most common mistakes when using Splice is incorrectly specifying the start index and the number of elements to remove. Be sure to double-check your code and ensure that you are specifying the correct index and delete count, or you may end up with unexpected results.
Not Accounting for Array Length Changes
When modifying arrays using Splice, keep in mind that the length of the may change. For example, if you are removing two elements from an of length 4, the resulting will have a length of 2. Be sure to account for these changes in your code to avoid errors.
Conclusion
As we have seen in this article, the Splice method is a powerful and versatile tool in JavaScript for modifying arrays. By using Splice to add, remove, and replace elements, developers can create dynamic and responsive applications that offer rich user experiences. By understanding the syntax and functionality of Splice, as well as common use cases and potential pitfalls, developers can optimize their use of this crucial JavaScript method.