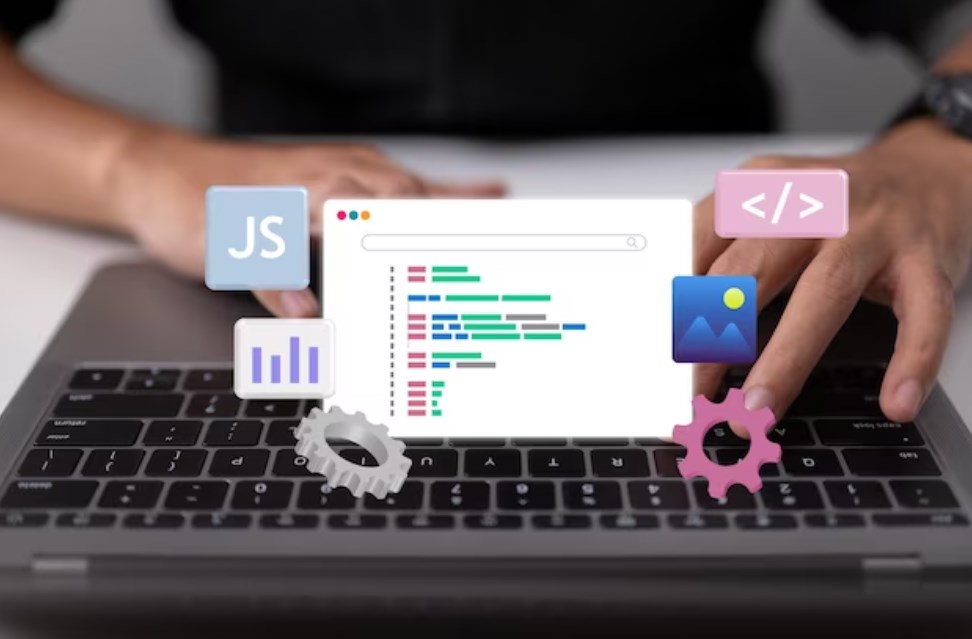
Navigating the intricate pathways of web development can sometimes be challenging. Among the many tools available for this endeavor is the Console in JavaScript—a foundational asset for debugging and logging.
This detailed guide will cover the console object’s numerous functionalities and explore how it can serve as an invaluable aid in troubleshooting, logging, and much more.
A Comprehensive Look at the JavaScript Console
The Console in JavaScript serves as a versatile instrument for web developers. It functions as both a debugging aid and a logging tool. It can show different kinds of information related to a particular script, from the states of variables to the outcomes of expressions and even the results of function invocations.
Moreover, it is also equipped to display warnings and errors, enhancing its utility as a comprehensive debugging tool.
Because the console object is globally accessible, it can be referred to in different ways such as window.console or simply console.
Sample Syntax
window.console.log("Greetings, world!"); // Using 'window'
console.log(123); // Global scope
console.log(10 * 5);
console.log(Math.PI);
Accessing the Console: Browser Shortcuts
The Console is accessible in various web browsers through specific keyboard shortcuts:
- Google Chrome: Utilize Ctrl + Shift + J on Windows or Cmd + Shift + J on a Mac.
- Mozilla Firefox: The shortcut is Ctrl + Shift + K on Windows or Cmd + Shift + K on a Mac.
- Microsoft Edge: One can open it with F12 or by pressing Ctrl + Shift + J.
- Safari: Initiate the console using Cmd + Opt + C.
Multifaceted Functions: Methods of the JavaScript Console
The Console object in JavaScript offers a plethora of methods, each contributing to better debugging and logging capabilities. Although the log method is the most commonly employed, there are several others, each with its unique utility.
List of Various Console Methods:
- console.log(): Logs general information;
- console.assert(): Tests conditions and logs messages;
- console.clear(): Clears the console;
- console.count(): Counts occurrences;
- console.dir(): Lists object properties;
- console.warn(): Outputs warnings;
- console.error(): Outputs error messages;
- console.table(): Displays tabular data;
- console.time(): Starts a timer;
- console.trace(): Provides a stack trace;
- console.group(): Groups related messages;
- console.info(): Gives informational messages.
The JavaScript Console is much more than a simple debugging tool; it is a multi-faceted instrument that can significantly enhance web development processes.
Whether you’re inspecting the state of variables, observing the results of expressions, or even monitoring the performance of function calls, the console object offers a variety of methods to assist you.
With global accessibility and a wide range of functionalities, understanding the Console is essential for anyone who takes JavaScript development seriously.
1. Console Logging
The console.log() function is among the most frequently employed debugging functions. It provides the means to display messages within the developer console.
This function has the capability to display a plethora of object types, encompassing strings, numerical values, booleans, arrays, HTML components, and more.
Usage:
console.log(123); // Numeric value
console.log("Greetings Universe!"); // Textual data
console.log(5 * 4); // Mathematical operation
console.log(new Date()); // Date object
// Several parameters
console.log("Hello", "Universe", 123);
2. Console Assertions
The console.assert() function evaluates a condition, and should the condition equate to false, it presents a specified message within the console. If the condition holds true, no action is taken.
Usage:
console.assert(condition, message, parameter1, parameter2, ...)
For the console.assert() function, at least two parameters are needed. The inaugural parameter is the condition being evaluated, followed by the designated message to appear should the condition be untrue.
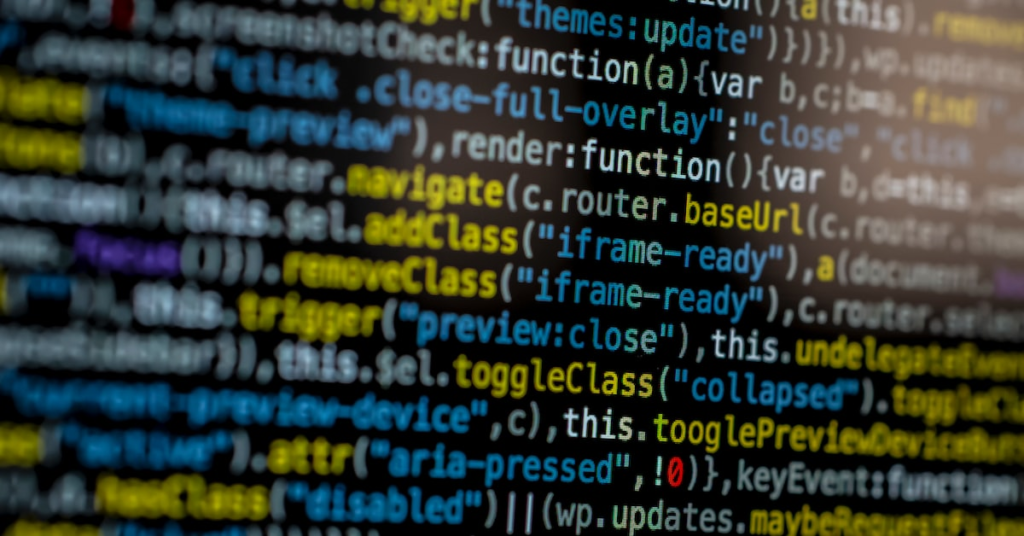
Illustrations:
console.assert(false, "This assertion failed.");
let integer = 10;
console.assert(integer > 20, "The integer value is under 20");
console.assert(25 === '25', "Mismatch in types", { "DataObject": 12345 });
3. Console Refresh
The console.clear() function has the purpose of refreshing the console, granted that the current environment allows for it.
This function proves handy when there’s a desire to refresh the console prior to logging fresh data. However, script-running environments like Node might remain unaffected.
Illustration:
console.log(10);
console.log("Hello Universe!");
console.clear();
console.log("Previous logs removed");
4. Console Tally
Utilizing the console.count() function, developers can keep a log on the frequency of function invocation.
This function can accept an optional label, which will be presented each time the function is invoked. Without a label, “default” is used.
Illustration:
for (let j = 0; j < 5; j++) {
console.count("Tally count");
console.log(j);
}
5. Console Directory Listing
With the console.dir() function, one can get a detailed, interactive enumeration of all the attributes associated with a designated object.
This gives a nested display of an object’s methods and attributes. It’s an effective tool to inspect all attributes of a given JavaScript object in a detailed manner.
Illustration:
const sequence = [1, 2, 3, 4, 5];
console.dir(sequence);
console.dir(document.location);
6. Console Error Messaging
The console.error() function is designed to produce error messages within the console. Such messages typically appear in a distinct red hue and are accompanied by an error icon.
It allows for customized error messages or object data to be displayed in scenarios where errors occur.
Usage:
console.error(parameter1, parameter2, ..., parameterN)
Illustration:
let value1 = 10, value2 = 0;
if (value2 !== 0) {
console.log(value1 / value2);
}
else {
console.error("Denominator is 0");
}
By incorporating these logging and debugging functions, developers gain a robust toolkit to navigate the multifaceted challenges of web development, ensuring that issues are swiftly identified and addressed.
7. Warning Message Display: The console.warn() Function
The console.warn() function is utilized to flag cautionary messages within the developer console. Such messages are highlighted in yellow, often accompanied by a yellow triangle sporting an exclamation mark to draw immediate attention.
- Color: Yellow
- Icon: Yellow triangle with an exclamation mark
- Utility: Best used to display cautionary messages or objects based on certain conditions.
Usage Pattern:
console.warn(condition_or_message)
Demonstration:
let figure = 99999;
if (figure > 10000) {
console.warn("Numeric value exceeds threshold");
}
8. Data Presentation in Tabular Format: The console.table() Function
The console.table() function offers an elegant way to represent data in a tabulated form within the console.
Mandatory Argument: Data, which should either be an array or an object.
Table Composition: Each array element transforms into a row in the table.
Indexing: For objects, the index column would represent property names, while for arrays it’s the actual numerical index of the elements.
Usage Pattern:
console.table(data)
Demonstration:
const alphabetArray = ["a", "b", "c", "d", "e"];
console.table(alphabetArray);
const individual = {
name: "Henry",
id: "X1YZ9",
age: 22
};
console.table(individual);
9. Code Execution Time Measurement: The console.time() Function
The console.time() function offers a handy way to assess the duration it takes for a snippet of programming logic to complete its execution.
- Initialization: A timer with a unique identifier is set up.
- Completion: console.timeEnd() is called with the same identifier to complete the timing process.
Usage Pattern:
console.time(timerName);
// Block of logic
console.timeEnd(timerName);
Demonstration:
console.time("loopTracker");
for (let i = 0; i < 10000; i++) { }
console.timeEnd("loopTracker");
10. Function Execution Tracking: The console.trace() Function
The console.trace() function serves the purpose of monitoring the execution flow of a particular function. This method is invaluable when one needs to understand the call hierarchy of a function—essentially, which functions are invoked in the process of its execution.
- Output: Produces a stack trace that shows the nested call structure for the function.
Usage Pattern:
console.trace();
Demonstration:
function alpha() {
console.trace();
}
function beta() {
alpha();
}
beta();
11. Consolidating Console Outputs: The console.group() Function
The console.group() function allows for the structuring of a batch of console messages into distinct sections, making the debugging process far more organized and manageable.
- Optional Argument: A label, which gets displayed as the group label;
- Termination: The group can be closed using console.groupEnd().
Usage Pattern:
console.group(groupLabel);
// Block of console messages
console.groupEnd();
Demonstration:
console.group("Collection One");
console.log("Hello");
console.log("Universe");
console.groupEnd();
console.group("Collection Two");
console.log("HTML5");
console.log("CSS3");
console.log("React");
console.groupEnd();
Through the use of these specialized console methods, debugging and data presentation within the development console can become a far more organized and insightful activity. These tools are invaluable for both novice and experienced developers seeking to understand and resolve issues within their codebases effectively.
12. Conveying Informative Notifications with console.info()
The console.info() function is specialized for communicating messages that carry informational weight. Essentially, it serves to annotate your development environment with insights that could be pivotal for debugging and code optimization.
- Argument: It takes a solitary argument, which is the message intended for display;
- Visual Indication: Accompanies the message with an “info” symbol to distinguish it from regular messages.
Usage Syntax:
console.info(target_message)
Illustrative Demonstration:
console.info("Delivering a message of informational nature");
Why Informational Messages Matter
Informational notifications can often serve as stepping stones for debugging more complex issues in the application. For instance, an info message can act as a flag to indicate a successful database connection or the retrieval of data from an API endpoint.
This permits developers to bypass scrutinizing these areas when looking for issues and focus their attention on potentially problematic segments of the code.
- Diagnostic Efficiency: Quick identification of code segments that are functioning as intended;
- Code Annotations: Serve as live comments within the code, aiding in rapid understanding of code functionality during reviews.
Situational Applicability of console.info()
While console.info() is pivotal in a debugging scenario, its usage isn’t universally applicable. For example, if a message needs immediate attention, console.warn() or console.error() might be more suitable.
Understanding the situational context for each type of console message is key to effective debugging.
- Risk Assessment: Different types of messages for varying levels of issue severity;
- Developer Communication: Info messages can also be used to communicate between team members who are working on different sections of the same project.
Performance Overheads and Best Practices
Although employing console methods like console.info() is often helpful during the developmental phase, it’s worth noting that they can lead to performance overheads, especially if overused.
It is generally recommended to remove or comment out such diagnostic commands before deploying the application to a production environment.
- Performance Impact: Multiple console commands can slow down browser performance;
- Best Practices: Removal or disabling of console commands during production deployment to preserve system performance and security.
Conclusion
The developer’s console in a JavaScript environment provides a robust set of tools designed to assist in various debugging and optimization tasks. Functions like console.info() play an essential role in facilitating smoother developmental workflows by offering valuable insights into code behavior and potential issues. However, it’s crucial to acknowledge that these console utilities are generally not intended for inclusion in production-level applications, as they can introduce both security vulnerabilities and performance issues.
Optimizing their usage can significantly expedite debugging processes, making it imperative for developers to understand each function’s unique capabilities and best practices. So, integrating these console functionalities can elevate the development process, contributing to more efficient and streamlined code management.