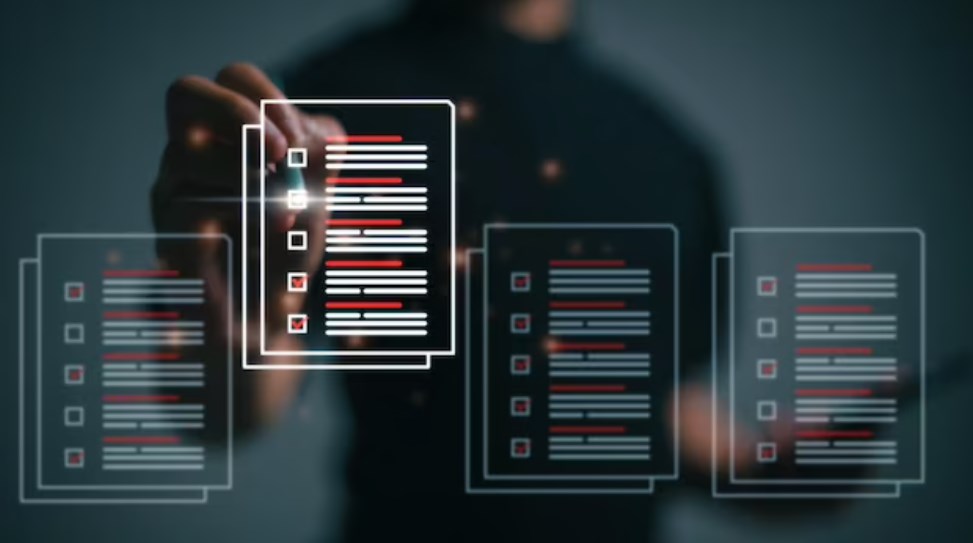
Are you looking for a way to extract checked options from checkboxes in JavaScript? This comprehensive guide dives deep into various techniques, elucidating how to retrieve the selected options in a form effectively.
From grouping by attribute name to using CSS selectors, we’ve got it all covered for you. This information-rich article is packed with authentic examples and scripts, aiming to elevate your JavaScript expertise.
1. Understanding the Basics: Checkbox and Its Importance in Forms
Checkboxes serve a vital role in data collection on the web, especially in forms where users may have to select more than one option. These rectangular boxes allow you to either opt in or opt out of multiple choices. Contrary to radio buttons, checkboxes facilitate multi-selection.
In HTML, a checkbox is defined as an input element with the type attribute set as “checkbox.” Each checkbox is usually accompanied by a “name” attribute for grouping and a “value” attribute to store its corresponding data.
<input type="checkbox" name="topics" value="Science">
Here, the “name” attribute enables the categorization of checkboxes, making them easier to manage.
2. How to Select Grouped Checkboxes Using Attribute Names
To perform group selection on checkboxes, you can leverage the attribute “name.” The JavaScript method document.getElementsByName() proves to be quite useful for this purpose, returning an array-like object comprising elements with the specified attribute name.
Example Script
<!-- HTML Structure --> <p>Select your areas of interest:</p> <input type="checkbox" name="area" value="Art"> Art<br> <!-- ... --> <button onclick="selectAllAreas()">Select All</button>
// JavaScript Logic function selectAllAreas() { let boxArray = document.getElementsByName('area'); let selectedValues = []; for (let idx = 0; idx < boxArray.length; idx++) { boxArray[idx].checked = true; selectedValues.push(boxArray[idx].value); } alert(selectedValues); }
In the above script, all the checkboxes belonging to the “area” group get selected upon clicking the button.
3. Utilizing querySelectorAll() to Achieve the Same Outcome
Another approach to batch-select checkboxes by group is the querySelectorAll() method. This function returns a NodeList, making it possible to perform operations similar to arrays.
Sample Code
let groupBoxes = document.querySelectorAll('input[name="area"]'); let pickedValues = []; for (let idx = 0; idx < groupBoxes.length; idx++) { groupBoxes[idx].checked = true; pickedValues.push(groupBoxes[idx].value); } alert(pickedValues);
This JavaScript script employs querySelectorAll() to achieve the same selection mechanism as before.
4. Gathering Selected Checkbox Data for User Choices
After the user has made their selections using checkboxes, the next step involves collecting this information for further processing. There are primarily two methods to achieve this:
A. Using the checked Property
The checked property returns a Boolean value indicating whether a checkbox is selected.
B. Employing CSS Selector :checked
Another way is to use the CSS selector :checked to filter the selected checkboxes.
Example Demonstration
let allBoxes = document.querySelectorAll('input[name="area"]'); let chosenValues = []; for (let idx = 0; idx < allBoxes.length; idx++) { if (allBoxes[idx].checked === true) { chosenValues.push(allBoxes[idx].value); } } alert(chosenValues);
Here, the checked property is employed to filter out and collect only the selected checkboxes from the group.
By following these methods and examples, you’ll be well-equipped to manage checkboxes and retrieve their selections proficiently in JavaScript.
Managing Checkbox Elements in Web Applications
- Selecting Your Preferred Programming Language: To work effectively, one must opt for a programming language that best suits their skill set. A typical choice among HTML, CSS, JavaScript, PHP, Python, or Ruby is often presented. After making your selection, simply press the indicated button to proceed with your choice.
- Employing the CSS Pseudo Selector :checked for Group Selection. Another alternative for choosing all activated selection squares within a particular group is by incorporating a specific CSS pseudo selector.
The :checked selector functions in CSS to target any selection square that is in an activated state.
With the help of the querySelectorAll() function, this CSS pseudo-selector can be employed to identify all selection squares within a given group.
Programmatic Illustration
// Identifying all activated selection squares within the ‘language’ group let activeBoxes = document.querySelectorAll(‘input[name=”language”]:checked’); // Retrieve the dataset from the active selection squares let extractedData = […activeBoxes].map(activeBox => activeBox.dataset); alert(extractedData);
Selecting All Checked Boxes Across an Entire Webpage
It’s possible to utilize an approach akin to the one discussed above to pick all selection squares across the entire website or application. The primary difference lies in the scope of targeting; instead of focusing on a particular group, this approach selects every checkbox present on the webpage.
The querySelectorAll() function can again be used for this purpose, but this time, the selector input:checked will target all selection squares that are active across the webpage.
Programmatic Illustration
// Targeting every active selection square across the document lets allActiveBoxes = document.querySelectorAll(‘input:checked’); // Gathering the dataset from the activated selection squares let allExtractedData = […allActiveBoxes].map(activeBox => activeBox.dataset); alert(allExtractedData);
Employing Event Listeners for Dynamic Interactions
Event listeners add an extra layer of interactivity, enabling real-time updates to the state of checkboxes. Using JavaScript functions like addEventListener, it’s possible to attach events that trigger specific actions when a checkbox is activated or deactivated.
For instance, enabling a “Select All” checkbox can be programmed to activate all checkboxes in a particular group.
Programmatic Illustration
// Applying an event listener to a “Select All” selection square document.getElementById(‘selectAll’).addEventListener(‘click’, function() { let groupBoxes = document.querySelectorAll(‘input[name=”group”]’); groupBoxes.forEach(box => box.checked = true); });
The Role of Web Accessibility
Accessibility is an often overlooked aspect when it comes to web forms and checkbox management. For those who rely on assistive technologies like screen readers, checkboxes should be clearly labeled and easy to navigate. Utilizing aria-label attributes or associating checkboxes with text through <label> elements can enhance accessibility.
Server-Side Checkbox Handling
While this guide has focused on client-side techniques, handling checkboxes efficiently often requires server-side logic. Backend languages like PHP or Python can read checkbox states sent via HTTP POST or GET methods to perform database updates or trigger specific actions.
Conclusion
This comprehensive guide has delineated various techniques to manage checkbox elements in web development, from choosing checkboxes in specific groups to selecting all checkboxes across an entire webpage. The methods presented are enhanced by utilizing CSS pseudo selectors and leveraging JavaScript’s powerful querySelectorAll() function.
For further optimization, dynamic event listeners and server-side logic can also be applied. This information aims to serve as a robust foundation for anyone seeking to master the manipulation of checkbox elements in web-based applications.