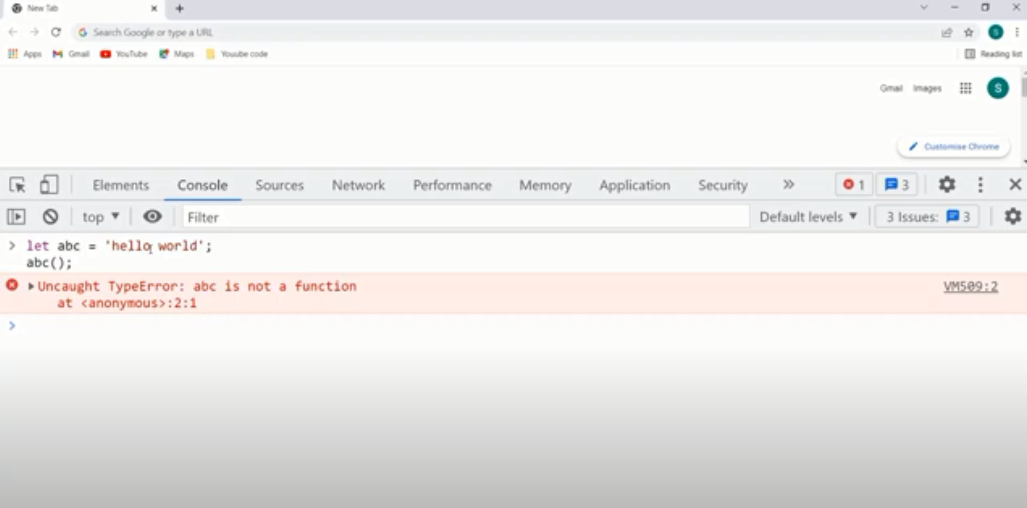
In the realm of JavaScript, a popular language for crafting web applications, manipulating strings is a common operation. One valuable method for this purpose is split(), which turns a string into an array of substrings using a provided delimiter. But what if you encounter an error stating ‘js split is not a function’?
This article will delve into this issue, elucidating what the split() method does, and how to prevent such errors.
Why Isn’t Split a Function in JavaScript?
JavaScript, a multi-purpose and extensively utilized language, offers numerous operations for handling strings in web application development. One such operation is the split() method, which divides a string into a collection of substrings based on a supplied delimiter.
Nonetheless, encountering an error message like ‘js split is not a function’ might leave you puzzled, questioning what led to this issue.
What Does the Split() Method Do in JavaScript?
The split() operation in JavaScript segments a string into a collection of substrings using a specified delimiter, returning an array holding these substrings.
Let’s look at the syntax for the split() method:
bash
string.split(separator, limit)
The mandatory separator is either a string or a regular expression defining the splitting parameters of the string. If the separator is omitted, the entire string becomes a solitary element in the resultant array.
On the other hand, the limit is optional and it’s an integer denoting the maximum split instances. Consequently, the array will hold no more than limit + 1 elements. If the limit is absent, the string is entirely split.
The split() method scrutinizes the original string for delimiters. Upon encountering a delimiter, it splits the string, adding a partial string to the resultant array. This procedure continues until the entire string is scanned.
Here’s an illustration of the split() method usage:
javascript
const str = 'Hello, World!';
const arr = str.split(','); // Split the string on commas
console.log(arr); // Output: ['Hello', ' World!']
In this scenario, the split() method splits the string str on the comma (‘,’) delimiter. The output array, arr, has two elements: “Hello” and ” World!”.
The split() operation is frequently used for activities like tokenizing strings, extracting string portions, or manipulating data separated by a certain character or pattern. It presents a handy method to dissect a string into smaller segments, enabling individual handling of each part.
Deciphering the Error
The error, “js split is not a function,” arises when an attempt is made to execute the split() method on a variable or object that is not of string type. It’s essential to understand that split() is a method specifically designed for strings, and its application on other data types like numbers, arrays, or undefined values is improper.
This error typically surfaces when there is a misconception that the targeted variable is a string, or when the variable value unpredictably alters during runtime. The resolution for this issue lies in verifying the type of the variable prior to invoking the split() method.
Rectifying the Error
To rectify the “js split is not a function” error, initiate by verifying the type of variable you’re trying to split:
- Utilize the type of operator to ascertain the type of the variable. In case it is not a string, evaluate why the variable possesses a different value than anticipated;
- Ensure to reassess the role of the variable, particularly if its value is dynamically modified during your code’s execution.
When dealing with user-supplied or external data, verify the input to ensure it adheres to your specifications. For instance, if a string is expected, employ conditional statements or regular expressions to affirm that the input aligns with the expected format. This precautionary measure aids in mitigating errors stemming from unanticipated data types.
One more potential cause of this error might be an issue with variable scope. Confirm that the variable you are trying to split is accessible in the current scope.
Alternate Solutions
In scenarios where the “js split is not a function” error is encountered and the variable in question is not a string, there are substitute methods to achieve the desired string manipulation:
A variable can be converted to a string using the String() function or the .toString() method. Post-conversion, the split() method can be safely employed on the string.
For more intricate string-splitting tasks, regular expressions paired with match() can be utilized. Regular expressions offer robust pattern-matching capabilities, enabling strings to be split based on diverse criteria like multiple characters, word boundaries, or even tailored patterns.
Final Thoughts
The “js split is not a function” error can be effectively addressed by ensuring that the variable on which the split() method is invoked is indeed a string. Confirm the variable type and review any user-provided or external data to avert unexpected errors.