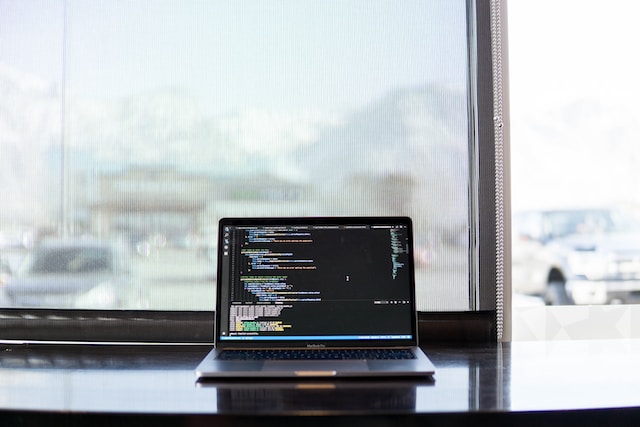
If you’re a JavaScript developer, then you know how frustrating it can be when your code isn’t working as expected. Luckily, JavaScript has several built-in tools that can help you troubleshoot your code and find and fix errors. One of these tools is the breakpoint, which allows you to pause the execution of your code at a specific point and inspect the state of your application. But did you know that there’s a JavaScript statement that works like a breakpoint? In this article, we’ll explore the benefits of using breakpoints in JavaScript and show you how to use them in your code.
Understanding Breakpoints in JavaScript
Before we dive into the details of the JavaScript breakpoint statement, let’s first take a closer look at breakpoints in general. A breakpoint is a tool that enables you to stop the execution of your program at a specific line of code. When you set a breakpoint, your program will pause at that line of code, allowing you to examine the state of the program at that point. This can be incredibly helpful in debugging your code, as it allows you to see what’s happening in your program and why it might not be working as expected.
What is a Breakpoint?
A breakpoint is a mechanism that enables you to pause the execution of code at a specific point. When a breakpoint is encountered, the execution of your program stops, and you can inspect the state of the program at that point. This is particularly useful when debugging your code, as it allows you to see what’s happening in your program and why it might not be working as expected.
Why Use Breakpoints in Debugging?
There are several benefits to using breakpoints when debugging your code. One of the main benefits is that it allows you to step through your code one line at a time, examining the state of the program at each step.
This can be incredibly helpful in understanding what’s happening in your program, and can help you identify and fix bugs more quickly and easily. Additionally, breakpoints can be used to set conditional breakpoints, which allows you to pause the execution of your program only when certain conditions are met. This can be particularly useful in complex applications where there are multiple paths that the code can take.
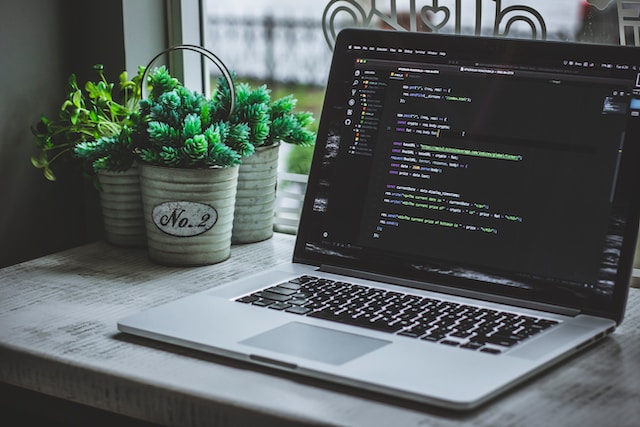
Alt: turned-on gray laptop computer
The Debugger Statement in JavaScript
The debugger statement is a JavaScript statement that works like a breakpoint. When the debugger statement is encountered, the execution of your program stops, and your debugger will be invoked. This allows you to examine the state of your program at that point and diagnose any issues that might be present.
Syntax and Usage
The syntax for the debugger statement is straightforward. Simply insert the statement at the point in your code where you want to set the breakpoint, like this:
function myFunction() { var x = 10; debugger; console.log(x);}
In this example, the debugger statement is inserted after the variable x is declared. When the code is executed, the program will pause at this line, and your debugger will be invoked.
Browser Compatibility and Support
The debugger statement is supported by all major browsers, including Google Chrome, Mozilla Firefox, Safari, and Microsoft Edge. However, it’s important to note that the use of the debugger statement is intended for debugging purposes only and should not be left in production code.
Breakpoints in Browser Developer Tools
If you’re working with JavaScript in the browser, you’ll be happy to know that all major browsers come equipped with developer tools that allow you to set breakpoints and debug your code. Here’s a quick overview of how to set breakpoints in the most popular browsers:
Setting Breakpoints in Google Chrome
To set a breakpoint in Google Chrome, open your developer tools by pressing F12 or Ctrl + Shift + I. Then, navigate to the Sources tab and find the file containing the code you want to debug. Once you’ve found the file, click on the line number where you want to set the breakpoint. A blue arrow will appear next to the line number, indicating that a breakpoint has been set.
Setting Breakpoints in Mozilla Firefox
To set a breakpoint in Mozilla Firefox, open your developer tools by pressing F12 or Ctrl + Shift + I. Then, navigate to the Debugger tab and find the file containing the code you want to debug. Once you’ve found the file, click on the line number where you want to set the breakpoint. A red dot will appear next to the line number, indicating that a breakpoint has been set.
Setting Breakpoints in Safari
To set a breakpoint in Safari, open your developer tools by pressing Option + Command + I. Then, navigate to the Sources tab and find the file containing the code you want to debug. Once you’ve found the file, click on the line number where you want to set the breakpoint. A blue arrow will appear next to the line number, indicating that a breakpoint has been set.
Setting Breakpoints in Microsoft Edge
To set a breakpoint in Microsoft Edge, open your developer tools by pressing F12. Then, navigate to the Debugger tab and find the file containing the code you want to debug. Once you’ve found the file, click on the line number where you want to set the breakpoint. A red dot will appear next to the line number, indicating that a breakpoint has been set.
Debugging with console.log()
While breakpoints are incredibly useful in debugging your code, they’re not the only tool at your disposal. Another useful debugging technique is using console.log(). This allows you to output information to the console, allowing you to examine the state of your program at different points in its execution.
Advantages and Disadvantages
One advantage of using console.log() over breakpoints is that you can output information to the console even when you don’t know where the bug is. This can help you narrow down where the bug might be located by outputting relevant information at strategic points in your code. However, one disadvantage of using console.log() is that it can be time-consuming to insert the statements throughout your code. Additionally, console.log() statements can clutter your console output, making it more difficult to spot other issues.
Tips for Effective Logging
When using console.log() for debugging, it’s important to keep a few tips in mind. First and foremost, be sure to label your console.log() statements clearly so that you know exactly what information is being output. Additionally, consider using console.group() and console.groupEnd() to group related output together, making it easier to read and understand. Lastly, be sure to remove any console.log() statements from your code before it goes into production, as leaving them in can potentially cause security issues.
Other Debugging Techniques in JavaScript
While breakpoints and console.log() are both incredibly useful in debugging your code, they’re not the only tools at your disposal. There are several other debugging techniques that can help you troubleshoot your code and find and fix errors. Here are a few examples:
Using the ‘debugger’ Keyword
The ‘debugger’ keyword is similar to the debugger statement we discussed earlier, but it can be inserted directly into your code rather than requiring you to open your debugger tools. When the ‘debugger’ keyword is encountered, your program will pause, and your debugger will be invoked. This can be incredibly useful in cases where you want to pause the execution of your code without setting a permanent breakpoint.
Profiling JavaScript Performance
JavaScript performance can be a major bottleneck in many applications, and profiling your code can be an effective way to identify areas where you can optimize your code for better performance. Many modern browsers come equipped with profiling tools that allow you to examine different aspects of your code’s execution, including CPU and memory usage.
Conclusion
Debugging your JavaScript code can be a time-consuming and frustrating process, but with the right tools and techniques, you can make the process go more smoothly. In this article, we’ve explored the benefits of using breakpoints in JavaScript and shown you how to use the debugger statement in your code. We’ve also shown you how to set breakpoints in different browsers, and demonstrated other debugging techniques such as console.log(), the ‘debugger’ keyword, and profiling JavaScript performance. By incorporating these tools and techniques into your workflow, you can become a more effective JavaScript developer and troubleshoot your code more efficiently and effectively.