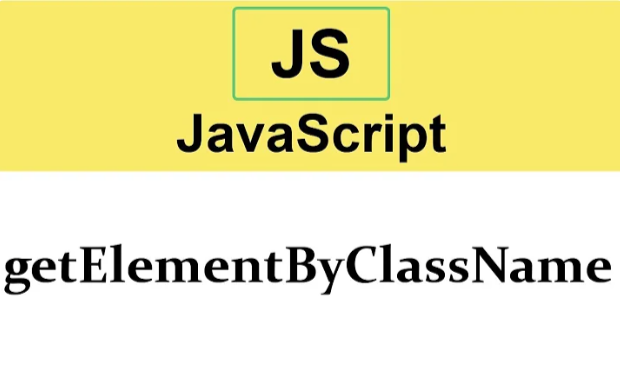
The constructor method in JavaScript plays a vital role in classes as it facilitates the creation and initialization of object instances. Typically, this method shares the same name as the class it is a part of. This characteristic presents a valuable opportunity to extract the class name of an object in JS by accessing its constructor name. This useful technique enables effortless identification and manipulation of objects based on their class association, contributing to enhanced object management and organization.
Understanding how to retrieve the class name of objects in JavaScript can significantly enhance debugging and development processes, ensuring more maintainable code. Explore further for its impact on SEO and link-building KPIs
The Class Name Attribute of JavaScript Objects
Unveiling the process of retrieving the Class Name of an Object in JavaScript, we first need to establish some foundational concepts.
- Class: Classes act as blueprints or templates that define the structure and behavior of objects. They provide a set of instructions for creating instances of a certain type;
- Object: Represents a specific entity that has its own unique state and behavior. It is an instance created based on a class, embodying the characteristics and functionality defined by the class;
- Constructor: The constructor is a special member function that is invoked using the new keyword when an object is being created. Its primary responsibility is to initialize the newly created object and assign initial values to its properties. The constructor sets up the object’s initial state, ensuring that it is ready for use and properly configured.
Building on these foundational concepts, let’s embark on a journey to explore multiple solutions for determining the Class Name of an Object in JavaScript. Through a series of insightful examples, we’ll delve into these solutions, providing a comprehensive understanding of how to achieve this essential task. Join us as we explore practical implementations and gain hands-on experience in working with Class Names in JavaScript Objects.
Solution 1: Constructor Function
When instantiating an object from a JavaScript class, the associated constructor function is called, either explicitly or implicitly. The primary goal of the constructor function is to initialize the object by assigning default or provided values. If a class does not have an explicitly defined constructor, the system automatically invokes a default constructor to ensure proper initialization of the object.
To retrieve the class name of an object, we can make use of the name property of the constructor function. By accessing this property, we can easily obtain the name of the class to which the object belongs. This feature provides a convenient and straightforward means to identify and manipulate objects based on their specific category names. Consequently, it enhances object management and manipulation capabilities, facilitating tasks such as dynamic type checking, organizing objects based on their class, or implementing conditional behaviors. Understanding and utilizing the class name property significantly contributes to efficient code development and maintenance.
This ability to access and utilize the class name allows developers to perform tasks such as dynamic type checking, implementing conditional behaviors based on the object’s class, or organizing and categorizing objects based on their class affiliation. It simplifies code maintenance and promotes modularity by providing a standardized approach to handle objects based on their class association.
Overall, the capability to retrieve the class name of an object in JavaScript through the constructor function’s name property empowers developers to efficiently manage and manipulate objects, facilitating cleaner and more organized code structures.
*Fact Check: It is important to note that the constructor is a function, whereas name is a property.
Syntax
[objectName].constructor.name
Let’s illustrate this concept with an example.
class Language {}
const l1 = new Language();
console.log(l1.constructor.name);
Output
Language
In the provided code snippet, we have defined an empty class named ‘Language’. Following that, we create an object of the class by invoking the constructor and storing the reference in the variable ‘l1’. Using the ‘new’ keyword, we instantiate an object of the “Language” class, utilizing its default constructor, ‘Language()’.
To retrieve the class name of the object, we can make use of the ‘obj.constructor’ function, which provides a reference to the constructor used for object creation. However, it’s important to note that this reference alone does not directly yield the class name.
To obtain the specific class name of the object, we need to access the ‘name’ property of the constructor function. This property holds the desired class name that we are seeking.
Solution 2: Function Inside Class
In JS, apart from directly accessing the constructor function, there is an alternative technique available for obtaining the class name of an object. This method involves creating a custom function within the class, specifically designed to return the object’s class name. By using the “this” keyword, which refers to the current object, we can access the “getClassName()” method and retrieve the class name associated with the object. This approach offers an added level of flexibility, enabling developers to implement custom logic within the function to retrieve and manipulate the class name according to their specific requirements. It provides an avenue for tailored class name retrieval and manipulation, expanding the range of possibilities for object management and customization.
Here’s an example to illustrate this approach:
Syntax
this.constructor.name
To better grasp this concept, let’s examine an example.
class Language {
getClassName() {
return this.constructor.name;
}
}
const l1 = new Language ();
const objClassName = l1.getClassName();
console.log(objClassName);
Output
Language
In the given example, we explore an alternative approach to retrieve the class name of an object in JavaScript. Instead of directly accessing the constructors, we utilize custom functions designed for this purpose. Specifically, we utilize the “getClassName()” function, which makes use of the built-in JavaScript constructor function.
By employing the “this” keyword within the “getClassName()” function, we can access the name of the current object within its scope. This technique allows us to dynamically retrieve the class name of the object, regardless of the specific constructor used.
This approach provides flexibility and abstraction, enabling developers to implement custom logic within the “getClassName()” function if needed. It allows for dynamic determination and manipulation of the object’s class name, expanding the possibilities for object management and customization.
To begin, we create an object of the “Language” class and store its reference. We then invoke the “getClassName()” method using the object reference.
*Fact check: This approach is relevant not only to objects created using a constructor function but also to objects created without one. These objects possess a “constructor” property that points to the corresponding Object constructor specific to their type. This property allows for easy identification and access to the constructor function associated with the object, regardless of how it was created. Hence, the technique remains applicable and useful in scenarios where objects are instantiated without the use of a constructor function.
Let’s examine the code provided below:
console.log([].constructor.name);
// Output: Array
Conclusion
In the world of JavaScript, there are two main techniques available for obtaining the Class Name of an Object. The first method involves creating an instance of a class and accessing the name property of its constructor method. Conversely, the second method revolves around creating a custom function that utilizes the this.constructor.name approach.
While both approaches yield the desired outcome of retrieving the Class Name of an Object, their suitability depends on the specific requirements of the problem at hand. The first method proves valuable when directly establishing the association between class and object types. On the other hand, the second solution shines by offering the flexibility to obtain class names through the implementation of custom functions. By considering the distinct strengths of each approach, developers can choose the most appropriate solution based on their particular needs and the overall design of their JavaScript application.