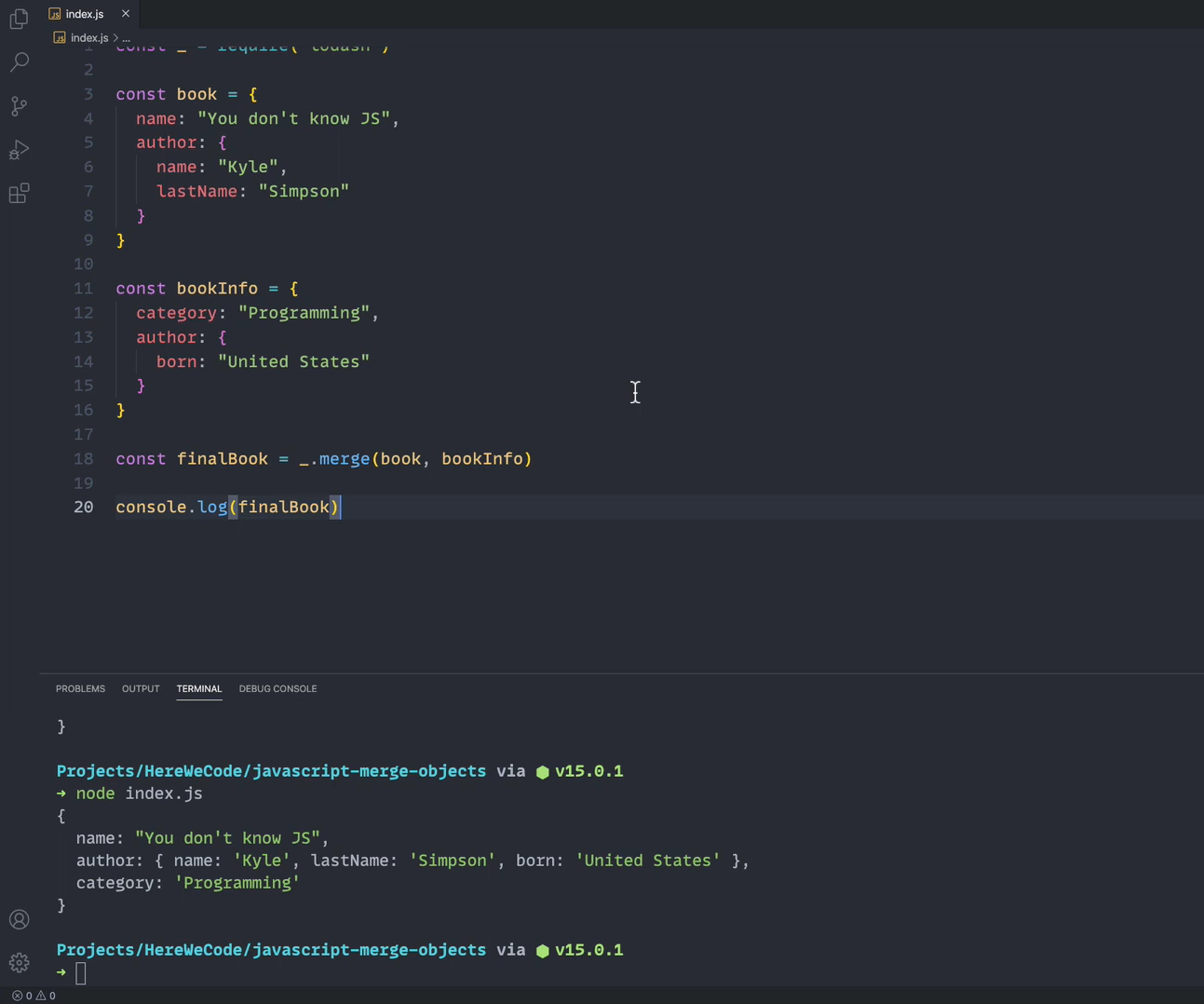
Alt: coding on the screen on neon purple background
Embark on an enlightening exploration as we delve into the world of merging objects in JavaScript. In this comprehensive article, we will not only uncover various approaches to merging objects but also provide practical examples to solidify your understanding. Merging objects is a common and fundamental task in JavaScript programming, allowing us to unify multiple objects into a harmonious whole by combining their properties.
Within the realm of JavaScript, we encounter two primary methods for merging objects: the shallow merge and the deep merge. Each approach offers its own unique benefits and considerations, which we will thoroughly explore. By the end of this article, you will have a comprehensive understanding of these merging techniques and be equipped with the knowledge to effectively merge objects in your JavaScript projects.
JavaScript Object Merging: Shallow Merge Explained
Shallow merging in JavaScript involves merging object properties without including any extended properties. In other words, the properties of the first object are overwritten with the corresponding property values from the second object.
The shallow merge can be achieved using the spread operator (…) or the ‘Object.assign()’ method. Let’s examine each of these methods in detail, accompanied by illustrative examples.
Using the Spread Operator (…) to Merge Objects
The spread operator (denoted as …) comes in handy when we want to merge objects while retaining the properties from both. It allows us to easily combine the elements of one object into another.
Let’s explore this method through an example:
let student = {
fName: ‘ABC’,
lName: ‘XYZ’,
sAge: 25,
rollNum: ‘SU1234’
};
let school = {
schoolName: ‘Excel Academy’,
location: ‘New York’
};
let Details = {
…student,
…school
};
console.log(Details);
Output
{
location: “New York”
fName: “ABC”
lName: “XYZ”
rollNum: “SU1234”
sAge: 25
schoolName: “Excel Academy”
}
When encountering identical property names, the property from the right-most object takes precedence and replaces the corresponding property in the merged object.
For Example
let student = {
fName: ‘ABC’,
lName: ‘XYZ’,
sAge: 25,
rollNum: ‘SU1234’,
location: ‘Washington DC’
};
let school = {
schoolName: ‘Excel Academy’,
location: ‘New York’
};
let Details = {
…student,
…school
};
console.log(Details);
Output
{
fName: “ABC”
lName: “XYZ”
location: “New York”
rollNum: “SU1234”
sAge: 25
schoolName: “Excel Academy”
}
In the given example, both the student and school objects have a property named “location.” When we merge these objects using the spread operator, the value of the “location” property from the school object replaces the value of the “location” property from the student object.
Using the Object.assign() Method to Merge Objects
Another method for merging objects in JavaScript is by utilizing the ‘Object.assign()’ method. This method copies all enumerable properties from a source object to a target object, ultimately modifying the target object. It then returns the updated target object.
Syntax
Object.assign(targetObj, …sourceObj)
Parameters (Required):
- targetObj: The object that will be returned after modification.
- sourceObj: Objects containing the properties to be merged.
Now, let’s explore how to merge objects using the ‘Object.assign()’ method, illustrated through an example.
let student = {
fName: ‘ABC’,
lName: ‘XYZ’,
sAge: 25,
rollNum: ‘SU1234’
};
let school = {
schoolName: ‘Excel Academy’,
location: ‘Washington DC’
};
let Details = Object.assign(student, school);
console.log(Details);
Output
{
fName: ‘ABC’,
lName: ‘XYZ’,
sAge: 25,
rollNum: ‘SU1234’,
schoolName: ‘Excel Academy’,
location: ‘Washington DC’
};
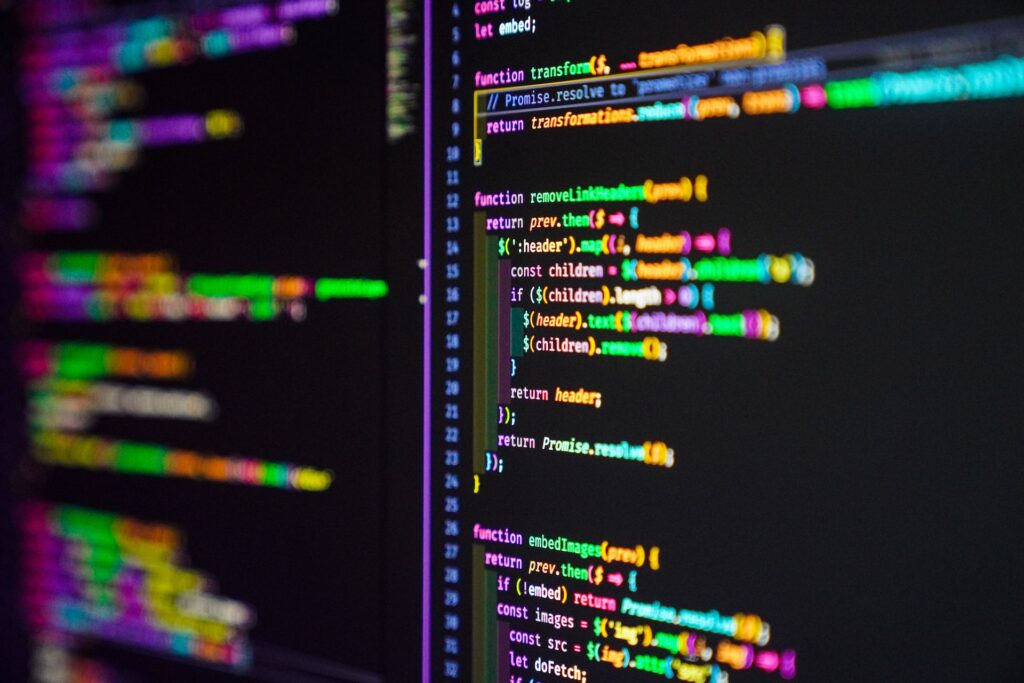
Deep Merging Objects in JS
When it comes to merging objects, the approach of shallow merging is suitable for combining first-level attributes. However, in scenarios involving complex objects with numerous nested levels or children, deep merging is highly recommended. Deep merging goes beyond superficial combination and ensures that all levels of the objects are duplicated, avoiding any references to the original objects. By employing deep merging, you can effectively merge intricate data structures and maintain the integrity of the merged result.
To achieve deep merging, we can utilize the ‘merge()’ method provided by the Lodash library.
The ‘_.merge()’ method merges objects from left to right, generating a new object with merged properties. When encountering identical keys, the resulting object will adopt the value of the right-most key. In the case of multiple identical objects, the newly created object will have a single key-value pair that accommodates those objects.
Syntax:
_.merge( objectName, sourceList )
Parameters:
- objectName: The destination object where the merged properties will be stored.
- sourceList: The source object(s) containing the properties to be merged. This parameter is optional and can accept multiple source objects.
Let’s grasp the concept better by exploring an example.
const _ = require(‘lodash’)
const iceCream = {
flavour: ‘Vanilla’,
addOn: {
sprinkler: ‘yes’,
topping: ‘chocoChip’,
},
}
const candy = {
name: “Blackcurrent”,
category: ‘candy’,
addOn: {
sprinkler: ‘yes’,
topping: ‘None’
},
}
const mergedValue= _.merge(iceCream, candy)
console.log(mergedValue)
Output
name: “Blackcurrent”,
addOn: {
sprinkler: ‘yes’
‘,
topping: “None”,
},
category: ‘candy’,
}
Consider that we have two objects, each with some common and distinct properties. To merge these objects, we can utilize the ‘_.merge()’ method. This method accepts the objects as parameters and returns the combined object as the output.
Conclusion
When merging objects in JavaScript, you have two main methods at your disposal. Shallow merging is ideal for simple objects with a single level of properties, while deep merging is better suited for complex objects with nested attributes and multiple levels.
From the examples discussed earlier, it is evident that deep merging is the preferred approach when dealing with intricate objects. Deep merging ensures that all levels of the objects are merged independently, without any references to the original objects. On the other hand, for simpler objects without nested attributes, the shallow merge provides a more straightforward and efficient solution. It’s important to choose the appropriate merging method based on the complexity and structure of your objects to achieve optimal results.