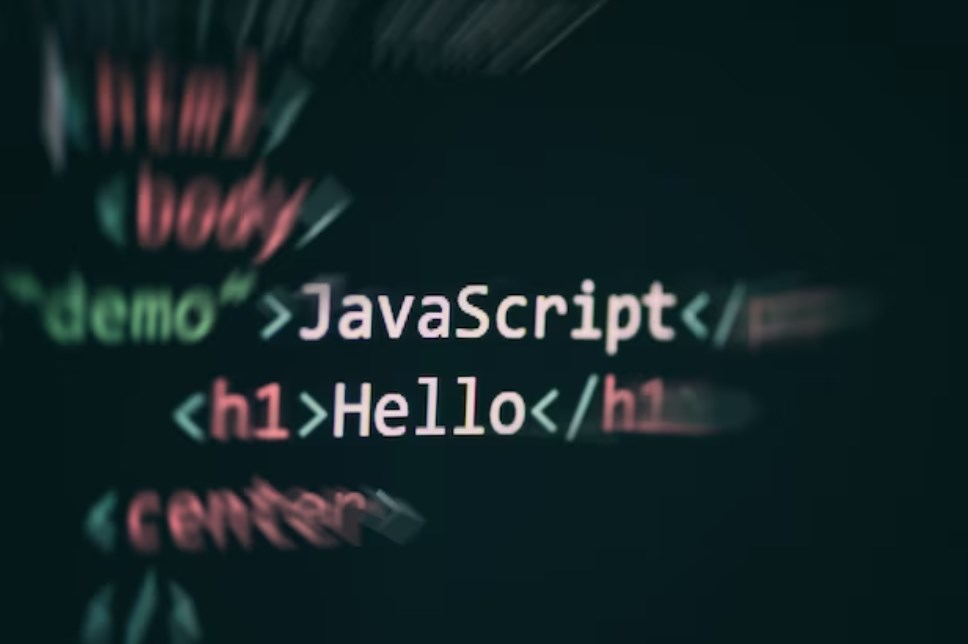
This comprehensive guide aims to provide a clear understanding of utilizing ‘for loops’ in JavaScript, but with a twist—we will focus on how to run them in reverse. If you’re eager to explore this less commonly used but incredibly useful technique, then this article is your go-to resource.
We will not only demystify the anatomy of a for loop but will also present multiple scenarios where reverse iteration can be of immense benefit.
What Is a For Loop in JavaScript?
The ‘for loop’ stands as one of the most versatile types of loops in the JavaScript language. It’s your go-to mechanism if the number of iterations is known beforehand. These loops have the capability to execute a sequence of statements multiple times, and they can work with various data types like arrays, strings, and objects.
- Primary Use: To iterate over a given block of instructions a specific number of times;
- Secondary Use: To loop through elements in arrays, characters in strings, properties in objects, and more;
- Sample: Forward Iteration
// Standard forward loop
for (let index = 0; index < 5; index++) {
console.log(index);
}
Most often, these loops progress from an initial point (like 0) up to a defined limit. But what if the task at hand calls for a backward loop?
Exploring Backward Loops in JavaScript
The mechanism to execute a loop in either direction actually resides in its very construct.
for (initialState; breakingCriteria; steppingValue) {
// Block of operations to be carried out
}
Here, the steppingValue section serves as the pivot around which the loop’s direction hinges. To traverse the loop backwards, the decrement operator comes into play.
- Attention: It’s important to note that when running a backward loop, initiating from the loop’s terminal point and modifying the breaking criteria are both necessary adjustments.
Example 1: Counting Downward in JavaScript
Let’s illustrate this by decrementing integers from 5 to 1.
// Backward iteration from 5 to 1
for (let counter = 5; counter > 0; counter--) {
console.log(counter);
}
Here, the loop commences at 5 and decrements, eventually ceasing at 1.
Example 2: Array Element Backward Traversal
As a more complex scenario, consider printing an array’s elements in reverse.
// Iterate backward over array elements
const numeralArray = [10, 20, 30, 40, 50];
for (let pos = numeralArray.length - 1; pos >= 0; pos--) {
console.log(numeralArray[pos]);
}
In this case, the array’s length property helps identify the terminal index, enabling the loop to proceed in a descending fashion through the array elements.
The use of length lets you start at the array’s last index and work your way backward, thanks to the decrement operation.
Running ‘for loops’ in reverse in JavaScript isn’t merely a programming curiosity; it’s a practical skill with direct applications in areas like data manipulation and algorithm optimization.
This article provides an overview of how to employ backward loops effectively, illustrated by rich examples and guided by best practices. With this knowledge, mastering the for loop in any direction should be within easy reach.
Example 3: Backward Iteration Through Strings
How about we traverse a string’s characters from its end to its beginning, using a for loop?
Utilizing the string’s length attribute gives us the uppermost index. To go through the characters in descending order, decrementing the index is the approach to follow.
Sample: Descending Iteration in Strings
// Outputting string characters from end to beginning
const greeting = "Hello";
for (let position = greeting.length - 1; position >= 0; position--) {
console.log(greeting[position]);
}
In this demonstration, the length attribute of the string assists in obtaining its uppermost index. With decrementing the index, the loop navigates from the string’s end to its beginning.
Benefits of Backward Looping
Exploring the merits of backward looping reveals why this method is advantageous in certain circumstances:
- Resource Optimization: When iterating backward through an array, specific operations become more efficient, especially when removing elements during the iteration;
- Algorithmic Applications: Certain algorithms, like sorting and searching techniques, may be optimized with backward iteration;
- Data Structure Navigation: For data structures like linked lists, backward looping can sometimes offer computational advantages.
Potential Pitfalls and How to Avoid Them
Like all techniques, there are things to watch out for when utilizing backward loops:
- Index Errors: Pay careful attention to the starting and stopping indices to avoid off-by-one errors;
- Readability: Code maintainability could be impacted; be sure to comment clearly when implementing backward loops;
- Performance: Although there can be performance gains, improper use might also lead to inefficiencies. Benchmark your loops to make sure you’re realizing the benefits you intend.
Real-world applications of Backward Looping
Understanding the practical applications of backward looping can give valuable context:
- Data Reversal: Whether it’s reversing strings for linguistic purposes or reversing arrays for computational tasks, backward loops are essential;
- Memory Conservation: In algorithms where the tail elements are more relevant than the head, using a backward loop can help in more efficient memory utilization;
- Buffer Operations: In low-level programming, buffering operations sometimes require data to be read or manipulated in reverse order, making these loops invaluable.
Conclusion
Backward looping in JavaScript with for loops provides an array of opportunities, from optimizing algorithms to efficient data manipulation. It’s a topic worth mastering for any coder looking to deepen their programming skillset.
Whether it’s array elements, string characters, or more advanced data structures, understanding how to iterate backward offers you a more robust set of tools for problem-solving in the coding world. Therefore, a thorough grasp of how to use this less conventional yet highly potent looping strategy is indispensable for modern programming challenges.