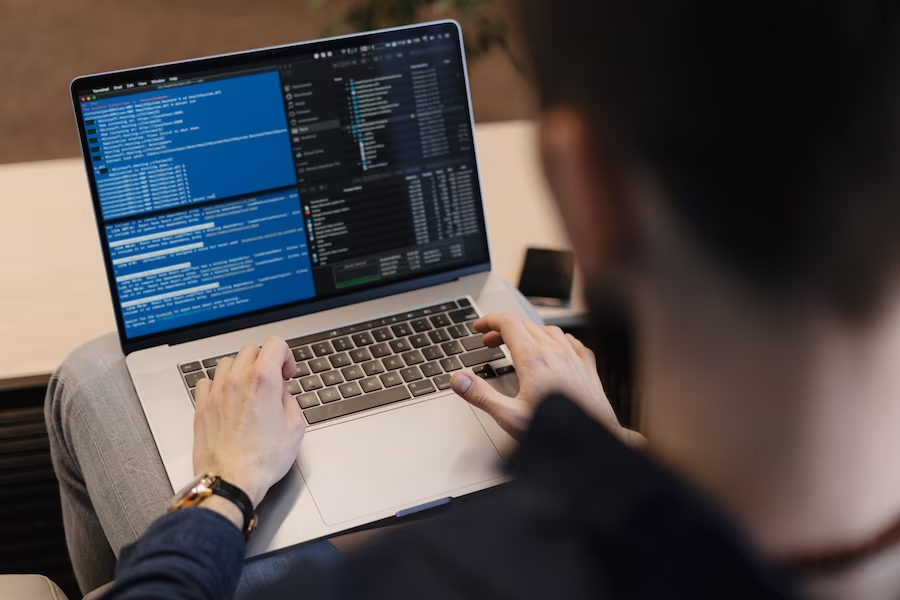
In programming languages, loops are a fundamental concept that enables the execution of a block of code multiple times based on specified conditions. JavaScript, a high-level, interpreted programming language, is no exception. It employs several types of loops, such as while, do-while, and for loops, among others.
JavaScript, a dynamic programming language, has a wealth of functionality that enables it to serve as the backbone of everything from basic scripts to large scale web applications. One of the essential tools in the JavaScript toolkit is the for loop, a vital construct in most programming languages.
The for loop is a control flow statement that allows code to be executed repeatedly based on a given Boolean condition. It has three parts: initialization, condition, and increment/decrement. Today, we delve deeper into a specialized form of the for loop – the nested for loop, which is essentially a for loop within another for loop.
The Anatomy of a For Loop
for (initialization; condition; increment/decrement) {
// code block to be executed
}
This is the basic syntax of a for loop in JavaScript. It’s straightforward and easy to understand once you become familiar with the three parts:
- Initialization: This part sets up the loop counter to an initial value. It’s where we declare our iteration variable, usually starting at a value of 0 or 1. The iteration variable typically goes by ‘i’, but it can be any valid variable name;
- Condition: This is a Boolean expression that the loop checks before each iteration. If it’s true, the loop continues; if it’s false, the loop stops. This part tests whether the loop should continue or not;
- Increment/Decrement: This part increments or decrements the loop counter. It’s usually a simple increment/decrement operation, but it can be more complex. This step occurs after the code block has been executed for a particular iteration.
A Basic Example of a For Loop
Let’s consider the following basic for loop that prints the numbers 1 to 5:
for (let i = 1; i <= 5; i++) {
console.log(i);
}
This simple loop illustrates all three parts of a for loop:
- Initialization (let i = 1): We declare a variable i and set its initial value to 1;
- Condition (i <= 5): This is the condition that the loop checks before each iteration. If i is less than or equal to 5, the loop continues; if not, the loop stops;
- Increment (i++): After each loop, we increment i by 1. This step occurs after the console.log(i) command is executed.
The code inside the loop, console.log(i), is executed for each iteration, printing the current value of i to the console. As a result, we get the numbers 1 to 5 printed.
Practical Uses of For Loops
For loops are used extensively in JavaScript for various purposes:
Array Iteration
A very common use case is to iterate over the elements in an array. Consider the following:
let fruits = ['Apple', 'Banana', 'Cherry'];
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
Here, we’re using a for loop to iterate over each element in the array fruits, printing each fruit name to the console.
Iterative Operations
For loops are excellent for performing operations a set number of times. For instance, if you wanted to generate the first ten numbers of the Fibonacci sequence, you could do so with a for loop.
let fib = [0, 1];
for (let i = 2; i < 10; i++) {
fib[i] = fib[i - 2] + fib[i - 1];
}
console.log(fib);
Variants of For Loops in JavaScript
In addition to the standard for loop, JavaScript offers a few variants that provide more concise, readable ways to iterate over data:
For-In Loop
This loop is used to iterate over the enumerable properties of an object in an arbitrary order.
let person = {firstName:"John", lastName:"Doe", age:25};
for (let key in person) {
console.log(key + ": " + person[key]);
}
For-Of Loop
This loop is used to iterate over iterable objects like arrays, strings, maps, and sets. It provides a simpler syntax when compared to traditional for loops.
let names = ["John", "Jane", "Joe"];
for (let name of names) {
console.log(name);
}
Diving Into JavaScript Nested For Loops
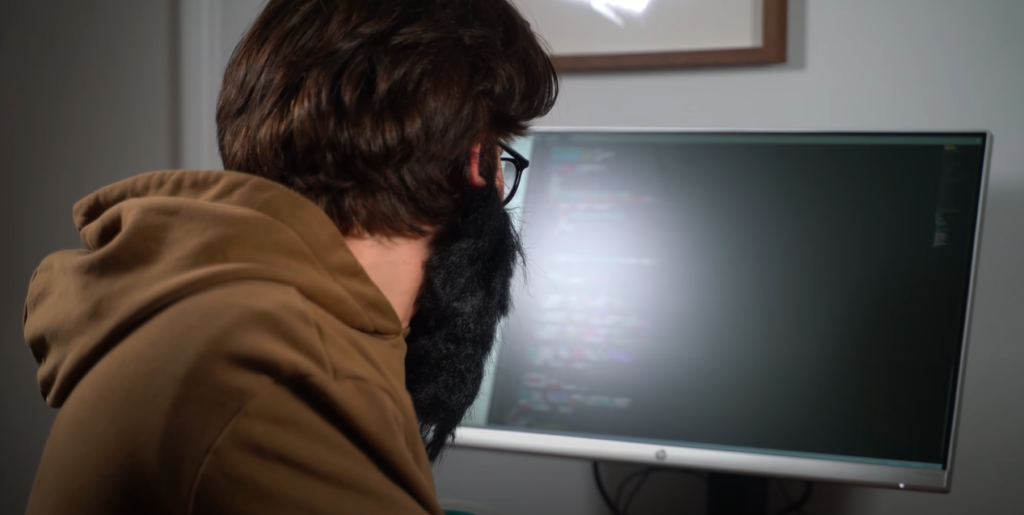
In JavaScript, a nested for loop is a loop within another loop. This means that for each iteration of the outer loop, the inner loop will run in its entirety. The structure of a nested for loop is as follows:
for (initialization; condition; increment/decrement) {
for (initialization; condition; increment/decrement) {
// code block to be executed
}
}
In this construct, the outer loop serves as the primary loop, while the inner loop is a secondary loop that’s executed within each iteration of the outer loop. For each cycle of the outer loop, the inner loop will run through its full cycle.
An Example of a Nested For Loop
Consider the following example of a nested for loop that prints a grid of numbers:
for (let i = 1; i <= 3; i++) {
let row = '';
for (let j = 1; j <= 3; j++) {
row += `${j} `;
}
console.log(row);
}
In this example, the outer loop (i) runs three times, indicating the creation of three rows. For each iteration of the outer loop, the inner loop (j) also runs three times, appending three numbers to each row. Therefore, the nested loop constructs a 3×3 grid of numbers.
Understanding the Flow of Control in a Nested For Loop
Understanding the flow of control in a nested for loop is critical. When the program encounters the outer loop, it checks the loop’s condition. If the condition is true, it enters the outer loop, where it encounters the inner loop.
At this point, the inner loop executes fully, meaning it runs through its initialization, condition check, block execution, and increment/decrement for as many times as the condition allows. Only once the inner loop’s condition becomes false does the program return to the outer loop’s increment/decrement statement.
This sequence repeats until the outer loop’s condition also evaluates to false. At this point, the program exits the outer loop and continues with the next line of code after the nested loop block.
When to Use Nested For Loops
Nested for loops come in handy in several scenarios in JavaScript programming:
let matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];
for (let i = 0; i < matrix.length; i++) {
for (let j = 0; j < matrix[i].length; j++) {
console.log(matrix[i][j]);
}
}
- Working with Multidimensional Arrays: Nested for loops are especially useful when dealing with multidimensional arrays. They allow you to iterate over each array and each element within those arrays;
- Creating Patterns: Nested for loops are also commonly used to create complex patterns or to construct grids, as we saw in the earlier example;
- Algorithms: Several algorithms, especially in computer graphics, game development, and simulations, often require the use of nested loops.
Performance Considerations for Nested For Loops
While nested for loops are a powerful tool, they do come with a cost – increased time complexity. Since each iteration of the outer loop causes the inner loop to run through its complete cycle, the total number of iterations can grow exponentially with the number of elements you’re working with. As a result, nested for loops can lead to slower performance with larger data sets.
It’s always crucial to use nested loops judiciously, considering alternatives if performance starts to become a bottleneck.
Use Cases of Nested For Loops
Below are some common use cases of nested for loops in JavaScript:
1. Accessing and Manipulating Multidimensional Arrays
A common use of nested for loops is to access and manipulate elements in multidimensional arrays (arrays within arrays). Consider the following example:
let arr = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];
for (let i = 0; i < arr.length; i++) {
for (let j = 0; j < arr[i].length; j++) {
console.log(arr[i][j]);
}
}
Here, the outer loop iterates over each sub-array, while the inner loop iterates over each element within the sub-arrays.
2. Creating Patterns
Nested for loops can be used to create various patterns. For instance, the following nested loop creates a simple pyramid pattern:
let n = 5;
for (let i = 1; i <= n; i++) {
let row = '';
for (let j = 1; j <= i; j++) {
row += '* ';
}
console.log(row);
}
This code generates the following output:
*
* *
* * *
* * * *
* * * * *
Pitfalls of Nested For Loops
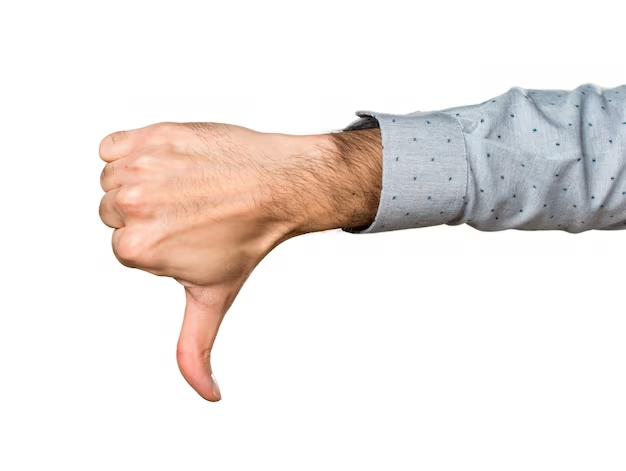
Nested for loops can be a powerful tool for iterating over multiple levels of data. However, they can also introduce challenges and pitfalls if not used carefully. In this discussion, we will explore some of the common issues associated with nested for loops, focusing on readability and complexity as well as performance concerns.
Readability and Complexity
One of the main drawbacks of nested for loops is their potential impact on code readability. When nested for loops are used, especially with multiple levels of nesting, the code can quickly become more complex and harder to understand. This complexity arises from the need to manage multiple loop counters and control flow logic. As a result, it becomes increasingly difficult to follow the code’s logic, making it harder to maintain and debug.
To illustrate this point, consider the following example with three levels of nested for loops:
arduino
Copy code
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
for (int k = 0; k < p; k++) {
// Perform operations on elements (i, j, k)
}
}
}
As the number of nested loops increases, the code’s indentation and complexity grow, making it challenging to comprehend the code’s purpose at a glance.
Performance Concerns
Another critical concern when using nested for loops is their impact on performance, especially when dealing with large datasets. The time complexity of a nested loop is determined by the product of the sizes of each loop. For instance, if we have two nested loops, each running ‘n’ times, the total number of iterations will be n * n (n-squared).
This exponential increase in iterations can lead to significant performance issues, as the execution time grows rapidly with the size of the dataset. For large datasets, the computational cost of nested for loops can become prohibitive, resulting in slow execution and inefficiency.
To mitigate the performance impact of nested loops, alternative algorithms or data structures can be considered. For example, techniques like memoization or dynamic programming can help optimize computations by avoiding redundant calculations.
Conclusion
Nested for loops in JavaScript are a powerful tool that can handle complex tasks, such as traversing multidimensional arrays or creating patterns. However, they must be used judiciously, considering their potential to introduce complexity and performance issues. By understanding these constructs, developers can harness their full potential while minimizing associated drawbacks.
FAQ
A nested for loop in JavaScript is a loop within another loop. The inner loop is executed entirely for each iteration of the outer loop.
Nested for loops are commonly used when working with multidimensional arrays and when you need to generate certain patterns or combinations.
Yes, often, nested for loops can be replaced with other constructs like map, reduce, filter, or forEach methods in JavaScript, especially when dealing with arrays.
Nested for loops can result in high time complexity, which can significantly slow down your program. Additionally, excessive use of nested for loops can lead to complex and hard-to-read code.
While technically there’s no limit to how deeply loops can be nested in JavaScript, it’s best to avoid deep nesting as it makes code hard to understand and can lead to performance issues.